This program is a demonstration of the use of C++ math header. Given 3 sides or angle values of a triangle, this program computes all 6 trigonometric ratios and print the result to the console.
The trigonometric ratios are calculated using builtin function from math.h header file.
Learn the basics of C programming before you try this example.
Problem Definition
The program gives two option to the user during run time. Depending on the available input values the user selects following.
- Find the 6 trigonometric values for a given angle.
- Find the 6 trigonometric values for given 3 sides.
If the user chooses the first option, then he or she must input the angle measure for which they wish to find the trigonometric ratio values.
The program than uses builtin trignometric functions from math.h header file such sin()
, cosine()
and tan()
functions to computes the output.
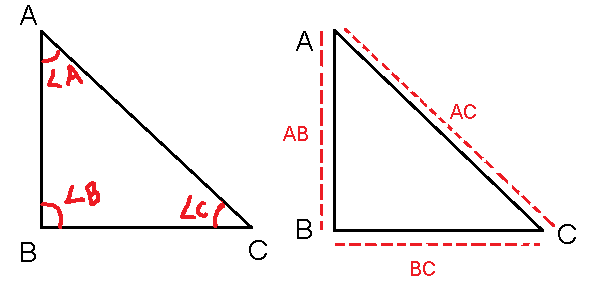
Secondly, if the user choice is finding trig rations using the length of sides of the triangle, then they must enter the values of sides.
First, enter the length of two shorter sides – AB and BC – where AB is opposite side and BC is the adjacent side of a triangle.
Then enter the length of the longer side (hypotenuse), but the value is not accepted if it is less than AB and BC because a hypotenuse is the longest side in a triangle.
The program computes the trigonometric ratios in the following manner.
\begin{aligned} &sin \hspace{3px}\theta = \frac {opposite \hspace{3px}side}{hypotenuse}= \frac{AB}{AC}\\\\ &cos \hspace{3px}\theta = \frac {adjacent \hspace{3px}side}{ hypotenuse} = \frac {BC}{AC}\\\\ &tan \hspace{3px} \theta = \frac {opposite \hspace{3px}}{ adjacent side} = \frac { AB}{AC}\\\\ &cosec \hspace{3px}\theta = \frac {1}{sin \hspace{3px}\theta}\\\\ &sec \hspace{3px}\theta = \frac {1}{cos \hspace{3px} \theta}\\\\ &cot \hspace{3px}\theta = \frac {cos \hspace{3px}\theta }{sin \hspace{3px} \theta} \end{aligned}
Program Source Code
#include <cstdlib>
#include <iostream>
#include <cmath>
#include <iomanip>
using namespace std;
int main()
{
float sine,cosine,tangent,cotangent,cosecant,secant;
float rad,degree;
rad = degree = 0.0;
int choice;
//sine=cosine=tangent=cotangent=cosecant=secant = 0.0;
cout <<"1. Find trig ratios using radian measure:" << endl;
cout <<"2. Find trig ratios using length of sides:" << endl;
cout <<"Enter your choice:";
cin >> choice;
if(choice == 1)
{
cout << "Enter the angle in degrees:";
cin >> degree;
rad = (degree/180) * 3.14;
sine = sin(rad);
cosine = cos(rad);
tangent = tan(rad);
secant = 1/cosine;
cosecant = 1/sine;
cotangent = cosine/sine;
}
else if(choice == 2)
{
float opposite,adjacent,hypotenuse;
cout << "Enter the length of each side:" << endl;
cout << "Length of opposite side(AB)?:" << endl;
cin >> opposite;
cout << "Length of adjacent side(BC)?:" << endl;
cin >> adjacent;
cout << "Length of hypotenuse (AC)?:" << endl;
cin >> hypotenuse;
sine = opposite/hypotenuse;
cosine = adjacent/hypotenuse;
tangent = opposite/adjacent;
secant = 1/cosine;
cosecant = 1/sine;
cotangent = cosine/sine;
}
else
{
cout << "Incorrect ! Choose correct option:" << endl;
}
//checking valid values for sin,cos,tan,cosec,sec,cot before printing
if(sine >= -1.0 || sine <= 1.0)
{
cout << "Sin" << " " << degree << "=" << sine << endl;
}
else
{
cout << "Sin" << "=" << "Undefined" << endl;
}
if(cosine >= -1.0 || cosine <= 1.0)
{
cout << "Cos" << " " << degree << "=" << cosine << endl;
}
else
{
cout << "Cos" << "=" << "Undefined" << endl;
}
if(degree != 90)
{
cout << "Tan" << " " << degree << "=" << tangent << endl;
}
else
{
cout << "Tan" << "=" << "Undefined" << endl;
}
if(degree != 90)
{
cout << "Sec" << " " << degree << "=" << secant << endl;
}
else
{
cout << "Sec" << "=" << "Undefined" << endl;
}
if(degree != 0 && cosecant <= -1.0 || cosecant >= 1.0)
{
cout << "Cosecant" << " " << degree << "=" << cosecant << endl;
}
else
{
cout << "Cosecant" << "=" << "Undefined" << endl;
}
if(degree != 0)
{
cout << "Cotangent" << " "<< degree << "=" << cotangent << endl;
}
else
{
cout << "Cotangent" << " " << "=" << "Undefined" << endl;
}
system("PAUSE");
return EXIT_SUCCESS;
}
Output
1. Find trig ratios using radian measure:
2. Find trig ratios using length of sides:
Enter your choice:1
Enter the angle in degrees:90
Sin 90=1
Cos 90=0.000796274
Tan=Undefined
Sec=Undefined
Cosecant 90=1
Cotangent 90=0.000796275
Press any key to continue . . .
If user choose the second option, then you get following results.
Enter your choice:2
Enter the length of each side:
Length of opposite side (AB)?:
5.6
Length of Adjacent side (BC)?:
7.8
Length of hypotenuse (AC)?:
10.55
Sin 0=0.530806
Cos 0=0.739336
Tan 0=0.717949
Sec 0=1.35256
Cosecant 0=1.388393
Cotantent = Undefined
Press any key to continue . . .