We know that pointers are used for an object of derived classes, we can also use them for the base objects. The pointer to the base class object is type-compatible (can be used in two ways) with a pointer to the object of the derived class.
To access the variable of the base class, a base class pointer will be used. So, a pointer is a type of base class, and it can access all, public function and variables of the base class since the pointer is of the base class, this is known as a binding pointer.
What is Pointer?
A pointer is a data type that stores the address of other data types in the original address of the memory location. We must declare a pointer before using it to store any variable address.
The general form of a pointer variable declaration is:-
data-type *variable name;
Example: int *emp;
The pointer of Base Class points to an unsimilar object of the derived class
Hence, To point an object to separate classes a single pointer variable is used.
What are Base Class and Derived Class?
Base Class: A base class is a class in OOP(Object Oriented Programming) from which other classes are derived. The Base class member functions and members are inherited from the Object of the derived class.
Base class member access specifiers derived in all three modes of inheritance in C++ are public, protected, and public.
The class which inherits the base class has all members of a base class as well as can also have some new additional properties. Another name for base class is parent class.
Derived Class: A class that is created from an existing class. The derived class can have more effectiveness concerning the Base class and can easily access the Base class.
The derived class inherits all members and member functions of a base class. Another name for Derived class is sub-class. It can inherit properties and methods of Base Class. All the code can be reused.
The syntax for creating Derived Class
class ExampleBaseClass{
// members....
// member function
}
class DerivedClass : public ExampleBaseClass{
// members....
// member function
}
Public inheritance
When a class uses public access specifier member to derive from a base, all public members of the base class are accessible as public members of the derived class and all protected members of the base class are accessible as protected members of the derived class.
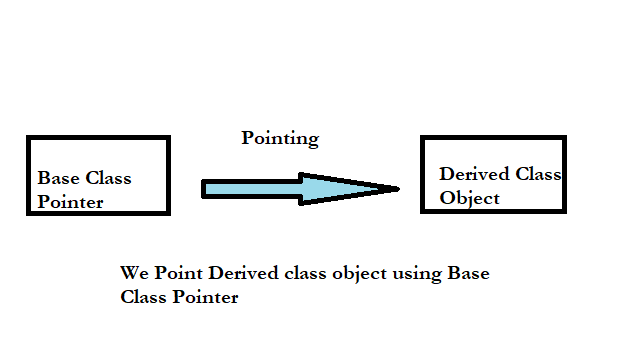
What is Upcasting?
Upcasting is converting a derived-class reference or pointer to a base class. In other words, upcasting allows us to treat a derived type as though it were its base type.
It is always allowed for a public inheritance, without an explicit typecast. This is a result of the is-a relationship between the base and derived classes
Although C++ permits a base pointer to point to any object derived from that base, The pointer cannot be directly used to access all the members of the derived class we may have to use another pointer declared as a pointer to the derived type.
A derived class is a class that takes some properties from its base class. A pointer of one class can indeed point to another class, but classes must be a base and derived class, then it is possible.
Variable of the base class can be accessed through a base class pointer will be used. So, a pointer is a type of base class, and it can access all, public function and variables of the base class since the pointer is of the base class, this is known as a binding pointer.
In this pointer base class is owned by the base class but points to the derived class object. Similarly, it works with derived class pointer, values are changed.
Example #1
#include<iostream.h>
class base
{
public:
int n1;
void show()
{
cout<<”\nn1 = “<<n1;
}
};
class derive: public base
{
public:
int n2;
void show()
{
cout<<”\nn1 = “<<n1;
cout<<”\nn2 = “<<n2;
}
};
int main()
{
base b;
base *bptr; //base pointer
cout<<”Pointer of base class points to it”;
bptr=&b; //address of base class
bptr->n1=23; //access base class via base pointer
bptr->show();
derive d;
cout<<”\n”;
bptr=&d; //address of derive class
bptr->n1=63; //access derive class via base pointer
bptr->show();
return 0;
}
Output:
The pointer of the base class points to it
n1 = 23
The pointer of base class points to derive a class
n1=63