Logical operator is one that compare two boolean operands and gives a boolean output. They are of high importance in computer science, especially in digital design and computer architectures.
They operands of logical operator could be constants, variables of all types and expressions involving comparison operators. First, we must understand the working of these logical operators.
Types of Logical Operators
There are three types of logical operators and they are:
Operator | Description |
&& | Logical AND operator |
|| | Logical OR operator |
! | Logical NOT operator |
Now, out of these three, you must be familiar with logical not operator which is also a unary operator. To learn more about unary operators visit following link.
Let us try to understand each logical operator separately.
Logical AND operator
The logical AND operator takes two or more operands and convert them to equivalent boolean value and return true if all of them are true, otherwise, if at least one operand is false, the result is false.
You can understand the working of this operator with the help of a truth table. A truth table gives output for all combination of variable inputs.
X | Y | Output |
T | T | T |
T | F | F |
F | T | F |
F | F | F |
The above is a two variable truth table for logical AND which shows that the output is true when all operands( X and Y) are true.
Example #1
//variable declaration
var x = prompt("Enter value for X:");
var y = prompt("Enter value for Y:");
var z = prompt("Enter value for Z:");
var real_x = Number(x);
var real_y = Number(y);
var real_z = Number(z);
//Check greatest of all
if (real_x > real_y && real_x > real_z)
{
alert("X is the greatest number");
}
else if ( real_y > real_x && real_y > real_z)
{
alert( "Y is the greatest number");
}
else if ( real_z > real_x && real_z > real_y)
{
alert("Z is the greatest number");
}
else
{
alert("Invalid Input! Numbers cannot be same");
}
Output #1
Logical OR operator
The logical OR operator takes two or more operands and convert them into their boolean equivalent. If either of the operands is true, the logical outcome is also true, otherwise it is false.
Here is the truth table for logical OR.
X | Y | Output |
T | T | T |
T | F | T |
F | T | T |
F | F | F |
In the above truth table, the outcome is false, when both operands (X and Y) are false. The rest of the entries are true.
Example #2
In this example, we will check if a number is within the range 1000 to 5000 including both numbers.
//variable declarations
var x = prompt("Enter your number:");
var real_x= Number(x);
//check range
if ( x >= 1000 || x <= 5000)
{
alert(" Valid Number");
}
else
{
alert("Invalid Number");
}
Output #2
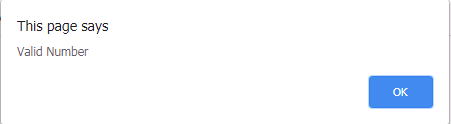
Logical NOT operator
The logical not operator takes a single operand and inverse the boolean value of it. Note than every literal or constant in JavaScript has a true or a false value by default. Most of them are true, except a few that are called falsy.
Here is the truth table for NOT operator.
X | Output |
T | F |
F | T |
Note that the number of rows corresponds to 2^n,where n is the number of operands.