In this article, you will learn about making a time management system which is developed using VB 6 and MS Access.
The system helps to do two simple task – Add new activity and View Activities based on following criteria.
- Important and Urgent
- Important and Not Urgent
- Not Important and Urgent
- Not Important and Not Urgent
The resultant view is based on the criteria selected.
System Diagram
The system diagram for time management has only three forms and a database. The added activity requires activity id, start date, end date, title and description for each activity. Also, you need to specify the criteria for each activity mentioned above.
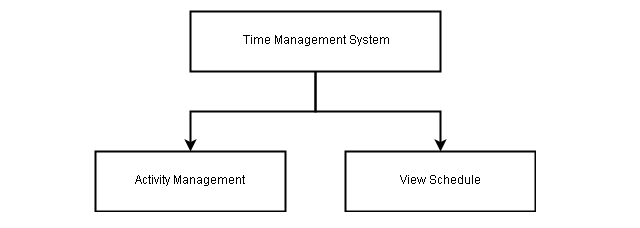
ER Diagram for Time Management System
The ER diagram contains the entity set and their relationship. The diagram contains a single entity – Activity.

The ER model will be converted into a relational model, discussed in the next section.
Relational Model and Table
The relational model is another representation of the ER model shown above. It is used to describe a schema for a relation or table.
Using the relational model we will make a table for our project. All the information regarding activities will be stored in a single table in the time management system.
Database and Table Creation
Our database for the project is created in MS Access. If you do not have MS Access then install any version above MS Access 2000. Create a new directory and call it AccessDB and also create a 2000-2003 database file and save it as a .mdb.
Open the .mdb database and go to Design View, a Save as dialog box will ask the name of the table, type Activity. You can create field name and data type as per the following diagram.
Now that the database design is complete, we must start the form design and coding in VB 6.
Form Design And Coding
The time management system has 3 forms and one module for database connectivity. In this section, we will discuss about designing the forms and coding for the controls that belong to each form.
- Time Management System (frmTimeManagement)
- Activity Management (frmActivityManagement)
- View Schedule (frmViewSchedule)
The time management system is the main screen and the entry point for the software. Activity management is where we manage activities. A user can :
- Add a new activity.
- Delete an activity.
- Modify an activity.
- View schedule based on importance and priority of the activities.
Form Time Management System
Components For Time Management
Form
Form Name: frmTimeManagement Caption: Time Management System Picture: Time.png (1024 x 680) Height: 9000 Width: 15000
Label
Lable Name: lblCopyright Caption: Copyright-Notesformsc.org BackColor: Window Text ForeColor: Highlight Text Height: 595 Width: 3354
Button
Button Name: cmdManage Caption: &Manage Style: 1-Graphical BackColor: Yellow (&H0000FFFF&) Height: 1173 Width: 3098 Top: 4330 Left: 6290
Code For Time Management
Private Sub cmdManage_Click() frmTimeManagement.Hide frmActivityManagement.Show End Sub
Form Activity Management
Components For Activity Management
Form
Form Name: frmActivitManagement Caption: Activity Management BackColor: Window Text ForeColor: Highlight Text Height: 9000 Width: 15000
Draw shapes and frames first and then other controls on top of it.
Shapes
Shape Name: Shape 1 BackStyle: 0-Transparent BackColor: Window Background (&H80000005&) BorderColor: Window Background (&H80000005&) BorderStyle: 1-Solid FillColor: Black (&H00000000&) FillStyle: 1-Transparent Height: 5895 Left: 600 Top: 1320 Shape: 0-Rectangle Width: 10455
Frames
Frame Name: frImportance Caption: Importance BorderStyle: 1-Fixed Single BackColor: Window Text ForeColor: Highlight Text Height: 2775 Left: 11400 Top: 1200 Width: 3135
Frame Name: frPriority Caption: Priority BorderStyle: 1-Fixed Single BackColor: Window Text ForeColor: Highlight Text Height: 2775 Left: 11400 Top: 4440 Width: 3135
OptionButtons
Name: optImportant Caption: Important BackColor: Window Text ForeColor: Highlight Text Height: 375 Left: 600 Top: 1080 Width: 2175
Name: optNotImportant Caption: Not Important BackColor: Window Text ForeColor: Highlight Text Height: 375 Left: 600 Top: 1680 Width: 2175
Name: optUrgent Caption: Urgent BackColor: Window Text ForeColor: Highlight Text Height: 375 Left: 600 Top: 1080 Width: 2175
Name: optNotUrgent Caption: Not Urgent BackColor: Window Text ForeColor: Highlight Text Height: 375 Left: 600 Top: 1680 Width: 2175
Labels
Label Name: lblActivityManagement Caption: Activity Management BackColor: Window Text ForeColor: Highlight Text Height: 495 Left: 4200 Top: 480 Width: 6015
Label Name: lblActivityID Caption: ActivityID BackColor: Window Text ForeColor: Highlight Text Height: 375 Left: 840 Top: 1440 Width: 1455
Label Name: lblStartDate Caption: Start Date BackColor: Window Text ForeColor: Highlight Text Height: 375 Left: 840 Top: 2400 Width: 1695
Label Name: lblEndDate Caption: End Date BackColor: Window Text ForeColor: Highlight Text Height: 375 Left: 840 Top: 3360 Width: 1695
Label Name: lblActivityTitle Caption: ActivityTitle BackColor: Window Text ForeColor: Highlight Text Height: 375 Left: 840 Top: 4320 Width: 2295
Label Name: lblActivityDesc Caption: Description BackColor: Window Text ForeColor: Highlight Text Height: 375 Left: 840 Top: 5280 Width: 2535
Text Boxes
Name: txtActivityID Text: 'leave empty' Height: 375 Left: 840 Top: 1800 Width: 2655
Name: txtStartDate Text: 'leave empty' Height: 375 Left: 840 Top: 2760 Width: 2655
Name: txtEndDate Text: 'leave empty' Height: 375 Left: 840 Top: 3720 Width: 2655
Name: txtActivityTitle Text: 'leave empty' Height: 375 Left: 840 Top: 4680 Width: 9855
Name: txtActivityDesc Text: 'leave empty' Height: 1335 Left: 840 Top: 5640 Width: 9855
Buttons
Name: cmdAdd Caption: &ADD Style: 1-Graphical BackColor: Yellow (&H0000FFFF&) Height: 615 Left: 600 Top: 7680 Width: 1935
Name: cmdDelete Caption: &DELETE Style: 1-Graphical BackColor: Yellow (&H0000FFFF&) Height: 615 Left: 3000 Top: 7680 Width: 1935
Name: cmdModify Caption: &MODIFY Style: 1-Graphical BackColor: Yellow (&H0000FFFF&) Height: 615 Left: 5400 Top: 7680 Width: 1935
Name: cmdView Caption: &VIEW Style: 1-Graphical BackColor: Yellow (&H0000FFFF&) Height: 615 Left: 10200 Top: 7680 Width: 1935
Name: cmdExit Caption: &EXIT Style: 1-Graphical BackColor: Yellow (&H0000FFFF&) Height: 615 Left: 12600 Top: 7680 Width: 1935
Code For Activity Management
Private Sub cmdADD_Click() Call loadcon Dim Impo As Boolean Dim NotImp As Boolean Dim Urge As Boolean Dim NotUrge As Boolean If optImportant.Value = True Then Impo = True End If If optImportant.Value = False Then Impo = False End If If optNotImportant.Value = True Then NotImp = True End If If optNotImportant.Value = False Then NotImp = False End If If optUrgent.Value = True Then Urge = True End If If optUrgent.Value = False Then Urge = False End If If optNotUrgent.Value = True Then NotUrge = True End If If optNotUrgent.Value = False Then NotUrge = False End If con.Execute ("INSERT INTO Activity(" & _ " ActivityID, StartDate, EndDate, ActivityTitle, ActivityDesc, " & _ " Important, NotImportant, Urgent, NotUrgent)" & _ " VALUES (" & txtActivityID & ", " & _ " '" & txtStartDate & "', " & _ " '" & txtEndDate & "', " & _ " '" & txtActivityTitle & "'," & _ " '" & txtActivityDesc & "'," & _ " " & Impo & ", " & _ " " & NotImp & "," & _ " " & Urge & "," & _ " " & NotUrge & ")") MsgBox ("Record Inserted") con.Close End Sub Private Sub cmdClear_Click() txtActivityID.Text = " " txtStartDate.Text = " " txtEndDate.Text = " " txtActivityTitle.Text = " " txtActivityDesc.Text = " " optImportant.Value = False optNotImportant.Value = False optUrgent.Value = False optNotUrgent.Value = False con.Close End Sub Private Sub cmdDelete_Click() Call loadcon con.Execute ("DELETE * FROM Activity WHERE ActivityID = " & txtActivityID & " ") MsgBox ("Task Deleted") con.Close End Sub Private Sub cmdExit_Click() Unload frmTimeManagement Unload frmActivityManagement Unload frmViewSchedule End Sub Private Sub cmdModify_Click() Call loadcon Dim Impo As Boolean Dim NotImp As Boolean Dim Urge As Boolean Dim NotUrge As Boolean If optImportant.Value = True Then Impo = True End If If optImportant.Value = False Then Impo = False End If If optNotImportant.Value = True Then NotImp = True End If If optNotImportant.Value = False Then NotImp = False End If If optUrgent.Value = True Then Urge = True End If If optUrgent.Value = False Then Urge = False End If If optNotUrgent.Value = True Then NotUrge = True End If If optNotUrgent.Value = False Then NotUrge = False End If con.Execute ("UPDATE Activity SET " & _ " StartDate = '" & txtStartDate & "'," & _ " EndDate = '" & txtEndDate & "'," & _ " ActivityTitle = '" & txtActivityTitle & "'," & _ " ActivityDesc = '" & txtActivityDesc & "'," & _ " Important = " & Impo & " ," & _ " NotImportant = " & NotImp & "," & _ " Urgent = " & Urge & "," & _ " NotUrgent = " & NotUrge & " WHERE ActivityID = " & Val(txtActivityID) & "") MsgBox ("Updated") End Sub Private Sub cmdView_Click() frmActivityManagement.Hide frmViewSchedule.Show End Sub
Form View Schedule – Time Management System
When you click the view button in the activity management , the view schedule form is shown and activity management form is hidden.
Components For View Schedule
Form
Form Name: frmViewSchedule Caption: View Schedule BackColor: Window Text ForeColor: Highlight Text Height: 9000 Width: 15000
Label
Label Name: lblViewSchedule Caption: View Schedule BackColor: Window Text ForeColor: Highlight Text Height: 615 Left: 4080 Top: 600 Width: 6855
Always create frames first and then other controls on top of it.
Frames
Frame Name: frImp Caption: Importance BackColor: Window Text BorderStyle: 1-Fixed Single Height: 2055 Left: 1080 Top: 1800 Width: 6135
Frame Name: frPriority Caption: Priority BackColor: Window Text BorderStyle: 1-Fixed Single Height: 2055 Left: 7680 Top: 1800 Width: 6135
Option Buttons
Name: optImp Caption: Important BackColor: Window Text ForeColor: Highlight Text Height: 375 Left: 720 Top: 720 Width: 3855
Name: optNotImp Caption: Not Important BackColor: Window Text ForeColor: Highlight Text Height: 375 Left: 720 Top: 1320 Width: 3855
Name: optUrgent Caption: Urgent BackColor: Window Text ForeColor: Highlight Text Height: 375 Left: 720 Top: 720 Width: 3855
Name: optNotUrgent Caption: Not Urgent BackColor: Window Text ForeColor: Highlight Text Height: 375 Left: 720 Top: 1320 Width: 3855
Buttons
Name: cmdSubmitQuery Caption: &Submit Query Style: 1-Graphical BackColor: Yellow (&H0000FFFF&) Height: 735 Left: 1080 Top: 7680 Width: 2775
Name: cmdClear Caption: &Clear Style: 1-Graphical BackColor: Yellow (&H0000FFFF&) Height: 735 Left: 4400 Top: 7680 Width: 2775
Name: cmdBack Caption: <-- Back Style: 1-Graphical BackColor: Yellow (&H0000FFFF&) Height: 735 Left: 7720 Top: 7680 Width: 2775
Name: cmdExit Caption: &Exit Style: 1-Graphical BackColor: Yellow (&H0000FFFF&) Height: 735 Left: 11040 Top: 7680 Width: 2775
Data Grid
Name: DataGrid1 Height: 2895 Left: 1080 Top:4440 Width: 12735
Code For View Schedule
Private Sub cmdBack_Click() Unload Me frmActivityManagement.Show End Sub Private Sub cmdClear_Click(Index As Integer) optImp.Value = False optNotImp.Value = False optUrgent.Value = False optNotUrgent.Value = False con.Close End Sub Private Sub cmdExit_Click(Index As Integer) Unload Me Unload frmActivityManagement Unload frmTimeManagement con.Close End Sub Private Sub cmdSubmitQuery_Click(Index As Integer) Call loadcon rs.CursorLocation = adUseClient rs.CursorType = adOpenStatic If optImp.Value = False And optNotImp.Value = False And optUrgent.Value = False And optNotUrgent.Value = False Then MsgBox ("Select Importance and Priority ! Try again") Unload Me frmActivityManagement.Show End If If optImp.Value = True And optUrgent.Value = True Then rs.Open "SELECT * FROM Activity WHERE Important = -1 AND Urgent = -1", con, adOpenDynamic, adLockPessimistic Set DataGrid1.DataSource = rs End If If optNotImp.Value = True And optUrgent.Value = True Then rs.Open "SELECT * FROM Activity WHERE NotImportant = -1 AND Urgent = -1 ", con, adOpenDynamic, adLockPessimistic Set DataGrid1.DataSource = rs End If If optNotImp.Value = True And optNotUrgent.Value = True Then rs.Open "SELECT * FROM Activity WHERE NotImportant = -1 AND NotUrgent = -1", con, adOpenDynamic, adLockPessimistic Set DataGrid1.DataSource = rs End If If optImp.Value = True And optNotUrgent.Value = True Then rs.Open "SELECT * FROM Activity WHERE Important = -1 AND NotUrgent = -1", con, adOpenDynamic, adLockPessimistic Set DataGrid1.DataSource = rs End If End Sub Private Sub Form_Load() optImp.Value = False optNotImp.Value = False optUrgent.Value = False optNotUrgent.Value = False End Sub
Module For Database Connectivity
A separate module is required to establish a connection with the database. This will enable access to the database and run query on it. In our project, the database is accessDB.mdb or the name you gave to your database earlier.
To create a module, go to Project Explorer > [right click] Modules < [mouse over] Add > [click] Module.
Add following code to the new module.
Public con As New ADODB.Connection Public rs As New ADODB.Recordset Public constr As String Public Sub loadcon() constr = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=C:\AccessDB\ActivityDB.mdb;Persist Security Info=False" con.Open constr End Sub
Note: The"C:\AccessDB\ActivityDB.mdb"
is the path to the database location. In your case, it might be different depending on where you store the MS access database file.