Extending a class in Java programming language is called inheritance. A parent class is created first and then it is extended using keyword – extends.
The child class inherits the properties and methods of the parent class and override them when necessary.
Problem Definition
In this example, we will create a parent class called the organization and declare a method called show(). Then we create another class that extends the organization class called branch. In the child class, you will override the method show() defined in organization class.
Program Code
class organization{
void show()
{
System.out.println("Organization");
}
public static void main(String[] args){
branch bx1 = new branch();
bx1.show();
}
}
class branch extends organization{
void show()
{
System.out.println("Branch Overrides");
}
}
Output
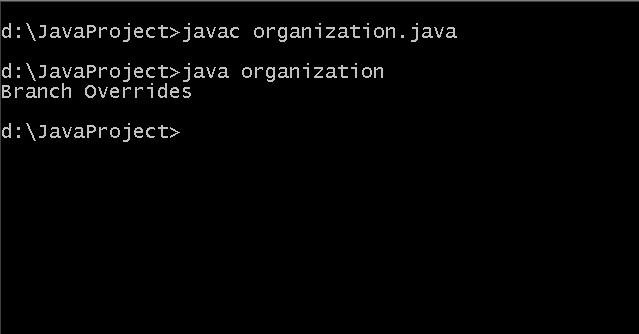