Some data types in python are mutable type which means you can make changes to them without changing the data type memory location, which immutable types do not allow changes to existing values in memory. Any change in variable value will be stored in a different location in memory.
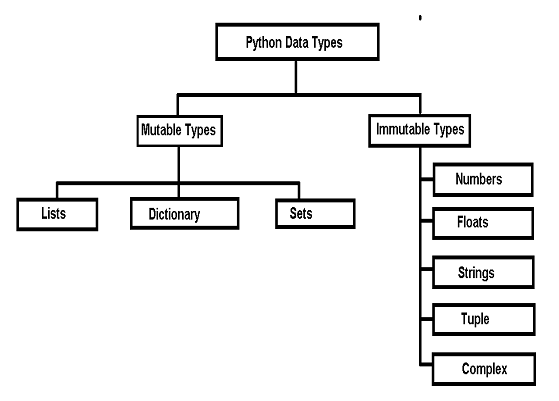
Here you will work on examples that demonstrate how mutable and immutable types work in python programming language. We recommend you to try the problem on your own first, then compare the results.
Program 1: Write a program that demonstrates working of immutable types.
Solution:
# Filename : Immutable_types.py
# Write a program that demonstrates working of immutable types
myInteger = 35
print("Before Integer Update",id(myInteger))
#update the integer
myInteger = myInteger + 100
#print the new value and its memory address
print(myInteger)
print("After Integer Update",id(myInteger))
myFloat = 15.45
print("Before Float Update",id(myFloat))
#update the floating number
myFloat = myFloat + 5.42
#print the new value and its memory address
print(myFloat)
print("After Float Update",id(myFloat))
myString = "Hello"
print("Before String Update",id(myString))
#update the string
myString = myString + "Radha"
#print the new value and its memory address
print(myString)
print("After String Update",id(myString))
myComplex = 2 + 5j
myComplexTwo = 4 + 2j
print("Before Complex Update",id(myComplex))
#update the complex numbers
myComplex = myComplex + myComplexTwo
#print the new value and its memory address
print(myComplex)
print("After Complex Update",id(myComplex))
myTuple = (34,66,77)
print("Before Tuple Update",id(myTuple))
#update the tuple
#Tuples cannot be changed , but if you convert a tuple to list then you can update it.
myChangeList = list(myTuple)
myChangeList[1] ="Superman"
myTuple = tuple(myChangeList)
#print the value and the memory address
print(myTuple)
print("After Tuple Update",id(myTuple))
Output:
Before Integer Update 1747543680
135
After Integer Update 1747545280
Before Float Update 43183456
20.869999999999997
After Float Update 42192624
Before String Update 43542976
HelloRadha
After String Update 43617040
Before Complex Update 42811048
(6+7j)
After Complex Update 42056088
Before Tuple Update 42121816
(34, 'Superman', 77)
After Tuple Update 42423680
Program 2: Write a program to demonstrate the working of mutable data types.
Solution:
# Filename : Mutable_type.py
# Write a program to demonstrate the working of mutable data types.
#sets
mySet = {23, 5, 66}
print("Before Set Update",id(mySet))
#update the sets using function update()
mySet.update("B")
#print the updated value
print(mySet)
print("After Set Update",id(mySet))
#lists
myList = [3, 6, 98, 22]
print("Before List Update",id(myList))
#update list by accessing the individual items
myList[1] = "Krishna"
#print the updated list
print(myList)
print("After List Update",id(myList))
#dictionary
myDictionary = {
"ID": 234,
"Name": "Mary",
"Subject": "Mathematics",
}
print("Before Dictionary Update",id(myDictionary))
#update the dictionary by accessing individual key
myDictionary.update({"Course":"BSc"})
#print the updated dictionary
print(myDictionary)
print("After Dictionary Update",id(myDictionary))
Output:
Before Set Update 44014208
{66, 5, 'B', 23}
After Set Update 44014208
Before List Update 42321400
[3, 'Krishna', 98, 22]
After List Update 42321400
Before Dictionary Update 43772976
{'ID': 234, 'Name': 'Mary', 'Subject': 'Mathematics', 'Course': 'BSc'}
After Dictionary Update 43772976
Observations: The mutable types do not have a different memory address after the update like the immutable types.