In this article, you will learn to write a C++ program to implement queue using pointers
A queue have two ends – front and the rear. The new elements are inserted from the rear position and deleted from front of the queue.
Problem Definition
We want to implement a queue using linked list structure. A single node contains data and a pointer link to the next node.
For example
struct node {
int data;
node *link;
};
The queue is initialize to NULL at the beginning with 3 defined operations on queue.
q.front = NULL;
q.rear = NULL;
// Three operations on Queue
void addq();
void delq();
void displayq();
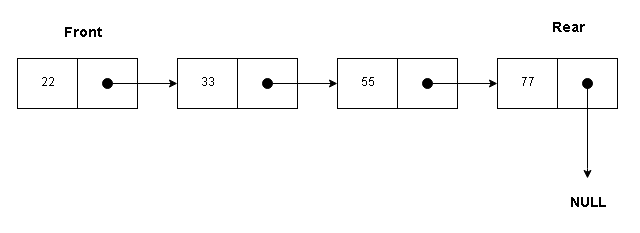
Program Code – Queue Using Pointers
/* C++ Program to Implement Queue using Pointers
File Name: Queue.cpp
Author: NotesforMSc
*/
#include "conio.h"
#include "iostream.h"
#include "malloc.h"
//Node structure
struct node {
int data;
node *link;
};
//Queue Structure
struct q1 {
node *front;
node *rear;
node *link;
};
//Queue Initialization
struct queue {
q1 q;
node *t;
public:
void initialize() {
q.front = NULL;
q.rear = NULL;
}
void addq();
void delq();
void displayq();
};
//Function Add Definition
void queue:: addq() {
t = new(node);
cout << "Enter Item To Add:";
cin >> t->data;
cout << "Item Added is:" << t->data;
t->link = NULL;
if((q.rear)== NULL)
q.front = t;
else
q.rear->link = t;
q.rear = t;
}
//Function Definition For delq()
void queue::delq() {
if(q.front == NULL) {
cout << "Queue Is Empty";
q.rear = NULL;
}
else {
t = q.front;
cout << "Item Deleted Is:" << q.front->data;
q.front=q.front->link;
free(t);
}
}
//Function Definition For displayq()
void queue::displayq() {
if(q.front == NULL) {
cout << " Queue Is Empty";
}
else {
cout << "\nFRONT";
for(t=q.front;t!=NULL;t=t->link)
cout << "-->" << t->data;
cout << "<--REAR\n";
}
}
//MAIN PROGRAM START HERE
void main() {
int choice;
queue qu;
clrscr();
qu.initialize();
cout <<"\n\t1-ADD";
cout <<"\n\t2-DELETE";
cout <<"\n\t3-DISPLAY";
cout <<"\n\t4-EXIT";
// Switch-Case for Menu
while(choice != 4)
{
cout << "\nEnter Your Choice:\t";
cin >> choice;
switch(choice) {
case 1:
qu.addq();
break;
case 2:
qu.delq();
break;
case 3:
qu.displayq();
break;
case 4:
break;
default:
cout << "Invalid Choice";
}
}
} //Main ends here
Output – Queue Using Pointers
1-ADD
2-DELETE
3-DISPLAY
4-EXIT
Enter Your Choice: 1
Enter Item To Add: 44
Item Added is: 44
Enter Your Choice: 1
Enter Item To Add: 55
Item Added is: 55
Enter Your Choice: 3
FRONT-->44-->55<--REAR
Enter Your Choice: 2
Item Deleted Is: 44
Enter Your Choice: 3
FRONT-->55<--REAR
Enter Your Choice: 4