In earlier lessons, you learned about try-catch-else-finally blocks. In this article, you will learn that we can put a try-catch block inside another try-catch block. Therefore, there can be more than one level of python nested try-catch blocks.
Understanding Nested Try-Catch
Each of these try-catch will handle different exceptions. Earlier we have seen exceptions at the same level. But, the objective of python nested try-catch is to find errors at different levels of code. See the figure below to understand how it works.
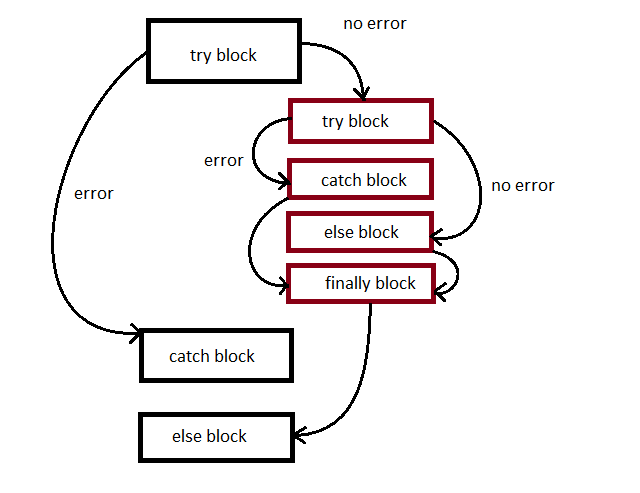
The program first checks the outer try-catch block
and if there is no exception, continues with the inner try-catch block.
The inner try-catch
block run independently and any exception or error is handled using inner catch block and all other blocks are executed sequentially.
Note that nested try block will not execute if outer try-catch
has an error.
Example #1 : Python Nested Try-Catch
In this example, we will receive amount, rate, and year as inputs and compute simple interest and total amount.
The user will enter the value and we want to make sure that there is no Type Error. Therefore, we will get the correct input.
Only after we receive correct input we must do compute the simple interest.
# check the correct input try: amount = int(input("Enter the amount: ")) rate = float(input("Enter rate in decimal, eg 0.12: ")) year = int(input("Enter number of Years: ")) simple_interest = 0.0 total = 0.0 # compute the simple interest try: simple_interest = amount * rate * year total = amount + simple_interest print("Simple Interest = ", simple_interest) print("Total amount", total) except Exception as err: print(err) else: print("Successfully Computed SI") finally: print("Completed!") except ValueError as err: print("Wrong type! try again later")
In the above program, we check input types and if the input types are not correct there will be an error and the exception is caught.
For example, user input 15000.42 as amount
which is a float value, however, the program is expecting an integer input. Therefore, it will cause a Value Error.
Enter the amount: 15000.42 Wrong type! try again later >>>
If the user put all values correctly, then the nested try-catch will be executed.
The output of the above is given below.
Enter the amount: 15000 Enter rate in decimal, eg 0.12: 0.9 Enter number of Years: 5 Simple Interest = 67500.0 Total amount 82500.0 Successfully Computed SI Completed! >>>
Note that all the other blocks such as else
and finally
also executed from the nested try-catch.
Example #2: Python Nested Try-Catch
Here we take a real world example, of file handling. Whenever, you try to manipulate a file. The first try-catch block will try to catch any file opening exceptions. Only after that file is opened successfully, nested try-catch is executed and catch other errors.
First step is to create a file named testFile.txt
and put some 4 or 5 lines of information.
Save the file in the same directory as the above program. Suppose the program name is file_m.py
, then you must put testFile.txt
in the same directory as file_m.py
.
Here is the code for the file_m.py.
# File Exception Handling with Nested Try-Catch try: file = open("testFile.txt","r") # print all file information to console try: for line in file: print(line) except Exception as error: print(error) else: print("Success") finally: file.close print("File closed") except (FileNotFoundError, PermissionError) as error: print(error)
The program above checks if there is a FileNotFoundError or a PermissionError happens. If file exists and there is no permission error. For example, suppose the file does not exist. Then you should receive error given below.
/File_Exception_Handling_With_Nested_try.py [Errno 2] No such file or directory: 'test.txt' >>>
The output of the above program is given below.
Orange Mango Banana Grapes Success File closed >>>
The nested try block will continue to manipulate the file and print contents of the file to the console. In the next article, we will discuss how exception handling is done with loops and control structures.