C programming language is a general-purpose high-level programming language and like every programming language, it follows a consistent structure. In this article, we begin the tutorial by describing the structure and elements of C language.
C Statements
A C program is consists of statements or instructions grouped together. A statement is an instruction to a compiler which usually consists of keywords describing the instruction.
For example, consider the following statement.
int rooms = 10;
In the above statement, keyword ‘int’ means integer and it used to describe a variable ‘rooms’ of type integer with a value 10.
printf ("My name is Thomas");
This statement does not contain anything other than keyword – printf.
The keyword, variable and the value consists of alphabets (a –z A-Z, digits (0 – 9), special symbols (=, #, %, ^ and many more).
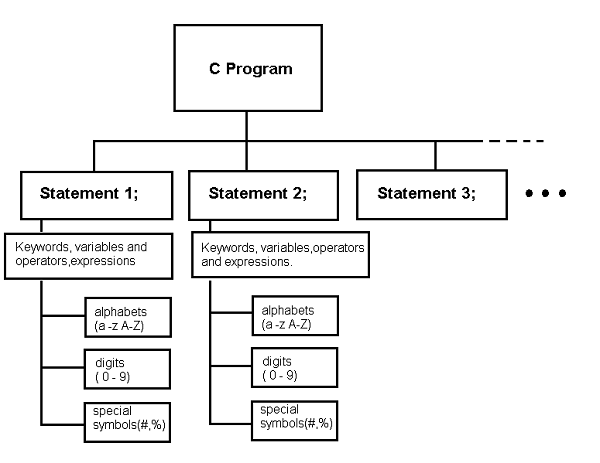
Note: Some statements do not have a variable or an expression and every C statement must end with a semi-colon.
Statement Block
Sometimes one or more C statements are blocked if they all contribute to one specific task. We put these statements between { and } brackets.
For example,
{
Statement 1;
Statement 2;
………………….
…………………..
Statement M;
}
A block of statement always starts with a keyword, which we will discuss in future lessons.
Nested Block
It is necessary to break a program into blocks of statements and break the block in sub-blocks to get clarity and efficiency in program logic. A block of statement which is inside of another block of statement is called a nested block.
For example, consider following diagram.
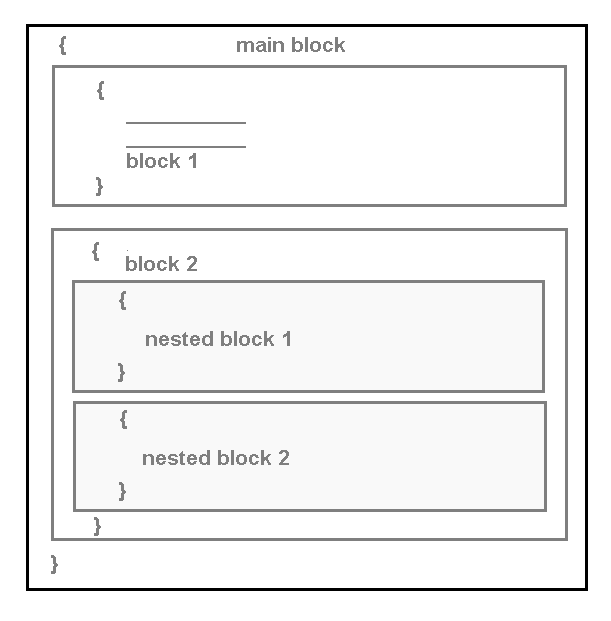
In the above program, there is a main block and two sub-blocks. The second sub-block has two nested blocks. The nested blocks can have more nested blocks if necessary.
First C Program
You can write a C program using any standard C compiler. Once you open the code editor add the following code into your compiler.
#include <stdio.h>
main ()
{
printf ("Hello World!");
return 0;
}
Every C program has a main block and inside the main block you can have multiple blocks or nested blocks of statements. A C program have following sections
- Global declaration
- Main function or block
- Main declaration
- Main program section
- Function definition section (optional)
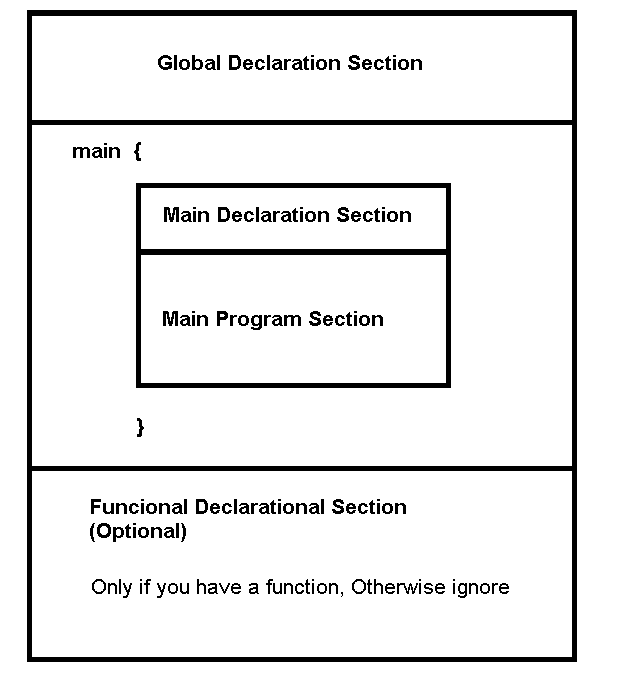
Global Declaration Section
In the global declaration section, we define the header file, global variable, declare functions. The header files are part of standard C library and if the program requires a library function then we must include the appropriate header file that contains the function.
For example,
#include <stdio.h> // if we want to use printf ();
Any variable or function that you define outside main has a global scope and can be used by other programs as well. These variables or functions must be declared in the global declaration section.
Main Program
The main () has the actual program code for a C program. It has two main section – main declaration section, main program section.
The main declaration section contains variables, functions declaration to be used in main program section. There scope is limited to the main (). The main () is a giant function or block which contains other blocks of statements.
Function definition section (optional)
A function is a block of code that does a specific task. The function definition section only required when you when you have declared a function in main () or global declaration section. Therefore, function definition will contain the actual statements for the function.
References
- Balagurusamy, E. 2000. Programming in ANSI C. Tata McGraw-Hill Education,.
- Brian W. Kernighan, Dennis M. Ritchie. 1988. C Programming Language, 2nd Edition. Prentice Hall
- Kanetkar, Yashavant. 20 November 2002. Let us C. Bpb Publications.