A string constant is an array of characters just like an array of integers. It helps to work with words and sentences in C programming. Yet, an array of integers and character array are not the same as strings.
Learn basic of C programming before you begin with strings. If you are familiar with the C basics continue reading.
What is a String constant?
A string constant is a one-dimensional array of characters with is terminated by , also called a null character.
char firstname[] = { 'P', 'E', 'T', 'E', 'R', '\0'};
The value of a character is
and each character in the string constant occupy
of memory. The syntax to initialize a string is very simple.
char name[] = "Rajesh";
/*where
char = data type of string.
name[] = indicates that it is a character array or a string.
" " = double quotes show that the value is a string.
Rajesh = actual string value.
*/
The C compiler inserts a automatically, so there is no need to specify
for every string that you create.
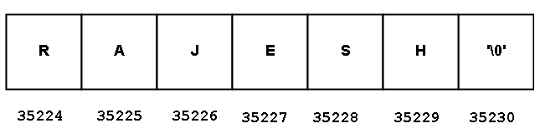
Reading and Printing String Constant
Reading input and writing out is an important part of the C programming language. To read a string constant use following syntax.
char name[10];
scanf("%s", &name)
You already know that is declared as a string of type char. The
is a builtin function that read inputs of various data types. To tell
that the input is a string use the modifier “%s”. But,
can only read a single word like “Rajesh”, and not “Rajesh Kumar”.
To read a multi-word string use or do following.
scanf("%[^\n]s", name);
The modifier is used for printing strings. For example,
printf("%s", name);
String Example program
It is time to implement everything you learned so far about strings. In this C program, you will perform two tasks.
- read and print a single word string.
- read and print a multi-word string.
Program Code
#include <stdio.h>
#include <string.h>
int main()
{
char input_name[35];
int choice;
/* Menu */
printf("Menu\n\n");
printf("1. Read Single Word\n");
printf("2. Read Multi-Word String\n");
printf("\n\n Enter your Choice:");
scanf("%d",&choice);
switch(choice)
{
case 1:
system("cls");
printf("Enter Single Word:");
scanf("%s", &input_name);
printf("%s", input_name);
break;
case 1:
system("cls");
printf("Enter Multi-Word:");
scanf("%[^\n]s", &input_name);
printf("%s", input_name);
break;
}
system("pause");
return 0;
}
Output
Menu
1. Read Single Word
2. Read Multi-Word String
Enter your Choice:1
Enter Single Word String:Paris
Paris
Press any key to continue . . .