In JavaScript, we have two sets of values – truthy and falsy. The main difference between these two are:
Truthy – Always returns true when done a Boolean comparison.
Falsy – Always returns false when done a Boolean comparison.
What are Falsy values?
The following is list of falsy
values:
undefined // variable is not having any values, returns false
null // same as undefined- no values and returns false
NaN // Not a number. NaN == NaN is incomparable.
0 //zero is falsy.
"" //empty string is falsy
false // false is a Boolean value that is by default false
Falsy and Truthy Concept
To understand the falsy
and truthy
concept consider the following example.
var A = "Mango";
if(A) // is true
{
console.log(A); // therefore, print A
}
The if
statement does not do any comparison, however, variable A
consists of a value which is not falsy
, therefore, returns true
.
Example Program: Remove Falsy from an Array
In this example, we will receive an array with values that also contains falsy. We will write a function that will remove all the falsy and returns the short array.
<!DOCTYPE html>
<html>
<head>
<title>JS: Check If A Value is Boolean</title>
<meta charset="utf-8">
<style>
main{
width:60%;
padding:2%;
margin:auto;
background:rgba(214,114,134,0.7);
text-align:center;
}
button{
max-width: 100%;
padding:1%;
background:red;
color:white;
}
#in{
width: 30%;
padding:1%;
margin:10px;
background:lightgrey;
color:black;
}
</style>
</head>
<body>
<main>
<h3>Remove all falsy from Arr = [1, null, NaN, 2, undefined]</h3>
<h2 id="out">Output Here</h2><br/>
<button onclick="extractFalsy()"> Remove Falsy</button>
</main>
<script>
function extractFalsy() {
// Don't show a false ID to this bouncer.
var arr = [1, null, NaN, 2, undefined];
var trueArr = [];
for(let i = 0;i< arr.length;i++){
if(arr[i])
{
trueArr.push(arr[i]);
}
}
document.getElementById("out").innerHTML = trueArr;
}
</script>
</body>
</html>
Output To Browser
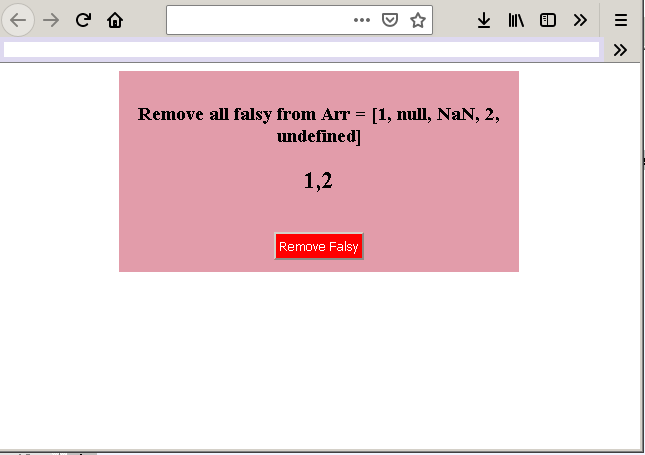