There are two category of HTML 5 elements listed below.
- Block level elements
- Inline elements
Block elements such as<mark style="background-color:rgba(0, 0, 0, 0);color:#b61515" class="has-inline-color"> </mark>
<h1> and <p> tags take the entire width of the document.
Inline elements such as <b> and <em>,<i>,<img> element will take just enough space within a block
element.
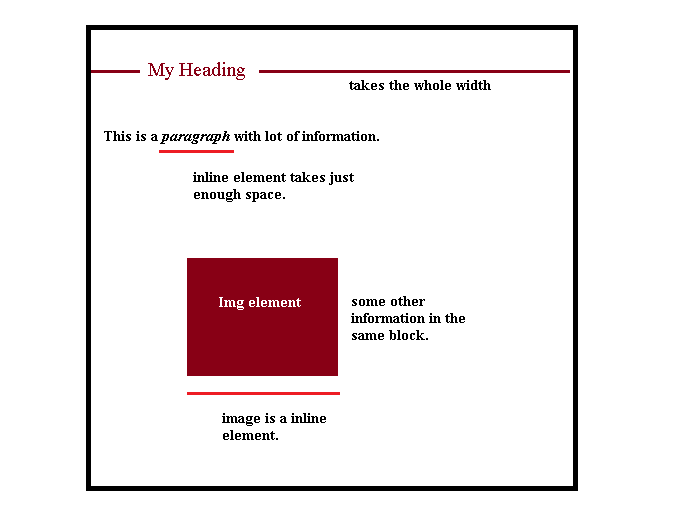
HTML 5 Semantic Element
There is no concept of document design in HTML. There is not structural meaning to the HTML elements.
Hence, Introducing HTML 5 semantic web elements and here is the list.
- <article>
- <aside>
- <details>
- <figcaption>
- <figure>
- <footer>
- <header>
- <main>
- <mark>
- <nav>
- <section>
- <summary>
- <time>
Each of these tags can define the content they hold.
JavaScript Data Types
JavaScript variables are container for values and can hold different types of data. Re-declaration is allowed, except in ECMAScript such as ES5, ES6 and so on. To declare a variable use following syntax:
var total = 234.80;
Now, we will check different types of variables.
//simple integer
var num = 34;
// floating point number
var secondNumber = 34.666;
//Strings
var name = " Peter Pan";
//You can use single quotes
var firstName = 'John';
When using a single quote or double quotes to enclose a string. Sometimes you need to use a single or double quote in the middle of the string. Use escape character (\”) or (\’).
var result = " You've Passed";
/*here not need to use escape character because double quote can enclose a single quote or single quote can enclose a double. Make sure inner quote is different from outer.*/
//Array
var arr = [34, 'tim', true, 33.77];
Array can contain different types of values. The array index starts with 0.
console.log(arr[0]);
//Will print the first element in the array.
//JavaScript Objects
//The JavaScript supports multiple type in the form of //key value pair.
//To declare a JavaScript object.
var car = {
"Model No": "Hundai",
"price": 2000,
};
To access an object property.
- Use dot notation. Example: Console.log(car.Price);
- Use bracket notation. Example: Console.log([‘Model No’]);
// store the property in a variable
var res = "Model No";
console.log(car[res]);
JavaScript Operators
Arithmetic Operators | Description |
+ | plus |
– | minus |
* | multiplication |
/ | division |
% | modulo |
++ | increment |
— | decrement |
Comparison Operators
Logical and Comparison Operators | Description |
> | greater than |
< | less than |
>= | greater than or equal to |
<= | less than or equal to |
!= | not equal to |
!== | strict not equal to |
== | equal to |
=== | strict equal to |
|| | logical or |
&& | logical and |
! | logical not |
How to Check Data Type in JavaScript?
To check the data type of any data element, use the typeof keyword.
var roll = 4455;
console.log (typeof roll);
//returns number
What will happen if combine number and string type?
console.log ("Str" + 44) ;
// output Str44
console.log(44 + 20 + "STR");
//output 64STR
The //output 64STR because JavaScript thinks that it a number and adds two number and when string comes.
Everything is converted to string. JavaScript accept the latest type available.