The For loop is another simple loop structure, which is different from other loops like Do loop and While Loop because it specifies a range before the loop could start.
The syntax for the For loop is given below.
Syntax
For index = start To end [Step step]
[Statements]
Exit For
[Statements]
Next index
Let us discuss the For loop structure.
For – keyword indicating the type of loop structure.
index – a variable that acts as index for the loop.
start To end – values that indicates start
of the loop and end
of the loop
Step step – The integer value that indicate how much to increment the loop at each iteration. Default is 1.
statements – some expressions or statements that the loop will execute at each iteration.
Exit For – immediately exit the For
loop.
Next Index – increment the loop index for next iteration.
Example Program: For Loop
In this example, we will create an index
for the loop and create a variable called sum
. Each iteration of the For loop, we will add a value of 10
to the sum
and display the results when the loop terminates.
Private Sub Command1_Click()
Dim sum As Integer, index As Integer
sum = 0
For index = 1 To 15
sum = sum + 10
Next index
MsgBox ("Sum is equal to" & " " & sum)
End Sub
In the code above the index is between 1 to 15
and each time it adds 10
to sum
. After the loop is terminated, the total amount of sum is display using a MsgBox
.
Output – For Loop
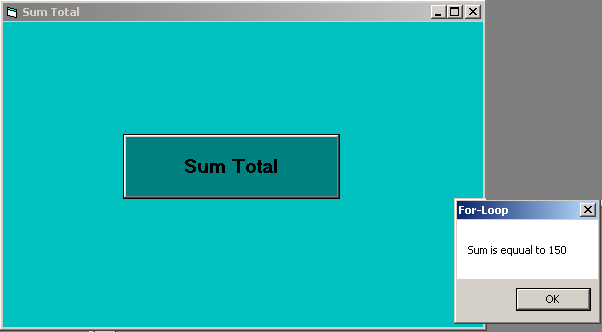