The program uses recursion to reverse an input number. A function is recursively called to extract each digit from the number and place it in reverse order. The final output is a reversed number. We wrote the program using Dev C++ version 4.9.9.2 on a Windows 7 64-bit system.
You must be familiar with following c programming concept before working on the example.
Problem Definition
The program receives an input number and reverse each by extracting the digits one at a time from original number n. It starts with two initial conditions. The remainder r = 0 and if n=0 then return 0.
The procedure to reverse the number 567 is shown below. The program does the following tasks to reverse the input number.
- Extract a digit from the unit position.
- Each iteration multiply the number with 10 to reverse it.
- Next extracted digit is added to previously obtained result.
- After final extraction the number is completely reversed.
Go through each step below to understand the working of the reverse() function.
Step 1: Extract the number 7
\begin{aligned}&r = r + n \hspace{2mm}\% \hspace{2mm}10\\ \\ &r = 0 + 567 \hspace{2mm}\% \hspace{2mm}10\\ \\ &t = 7 \end{aligned}
Step 2: Change the position of a number from unit place to tenth place.
\begin{aligned} &r = r * 10\\ \\ & r = 7 * 10\\ \\ &r = 70 \end{aligned}
Step 3: Get ready for the next extraction.
\begin{aligned} &= reverse (n/10)\\ \\ &= reverse (567/10)\\ \\ &= reverse (56) \end{aligned}
Step 4: Extraction the second digit when value of r = 70 from Step 2.
\begin{aligned} &r = 70 + n \% 10\\ \\ &r = 70 + 56 \% 10\\ \\ &r = 70 + 6\\ \\ &r = 76 \end{aligned}
Step 5: Change position of r to tenth place to hundredth place.
\begin{aligned} &r = r * 10\\ \\ &r = 76 * 10\\ \\ &r = 760 \end{aligned}
Step 6: Get ready for a new n value.
\begin{aligned} &= reverse (n/10)\\ \\ & = reverse ( 56/1)\\ \\ & = reverse (5) \end{aligned}
Step 7: Extract last digit from original number, when r = 760
\begin{aligned} &r = r + n \% 10\\ \\ & r = 760 + 5\\ \\ &r = 765 \end{aligned}
The number is successfully reversed.
Note:- The above steps are demonstration of reversing a 3 digit number, hence, the number of steps are limited. If you take a larger number there will be more steps.
Flowchart – reverse a number using recursion
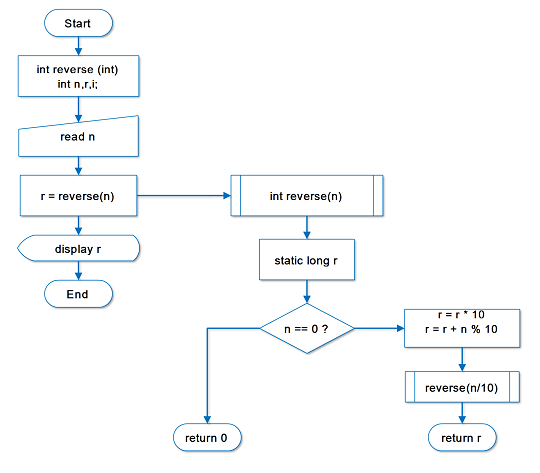
Program Code – reverse a number using recursion
#include
#include
int reverse(int);
int main()
{
int n, r, i;
printf("Enter a Number to Reverse:");
scanf("%d", & n);
r = reverse(n);
for (i = 0; i < 30; i++)
printf("_"); printf("\n\n");
printf("%d\n\n", r);
for (i = 0; i < 30; i++)
printf("_"); printf("\n\n");
system("PAUSE");
return (0);
}
/* The Reverse Function */
int reverse(int n)
{
static long r = 0;
if (n == 0)
{
return 0;
}
r = r * 10;
r = r + n % 10;
reverse(n / 10);
return r;
}
Output
The program ask for an input number, when you enter a number – for example, 244. The program reverses the digits of the number and prints on the console. The solution is 442.
Enter a Number to Reverse: 244
_______________________________
442
_______________________________