The C program to count the frequency of vowels, consonants and white spaces for a given word or a sentence is a string manipulation program. A C loop with conditions that checks the given input string and count the number of vowels, consonants, and white spaces.
We compiled this program using Dev-C++ compiler installed on a Windows 7 64-bit system. You may try another standard C compiler and to get an error free program make necessary changes to the source code.
Also, before you try this example, learn following C programming concepts. If you are familiar with them, continue reading.
- C Printing Outputs
- C Reading Input Values
- C Data Types
- C Logical Operators
- C Flow Control Structures
- C For Loop
Problem Definition
In C language every string is like an array with each character of the string hold a position in this array. The last character is null character by default.
Using this array logic, the program read a word or a sentence during execution and check each and every character of input string. It counts the number of vowels, consonants and white spaces.
For example, the array representation of word – SPIDERMAN as follows.
\begin{aligned} &0 \hspace{5px}1 \hspace{5px}2\hspace{5px} 3 \hspace{5px}4 \hspace{5px}5 \hspace{5px}6\hspace{5px} 7\hspace{5px} 8 \hspace{5px}9 \\ &S \hspace{3px} P\hspace{3px} I \hspace{3px}D \hspace{3px}E \hspace{3px}R \hspace{3px}M\hspace{3px} A \hspace{3px}N \hspace{3px}\backslash\empty\end{aligned}
Note:- The Null character is added to all the strings in C by default.
Flowchart – Program to Count Frequency of Vowels
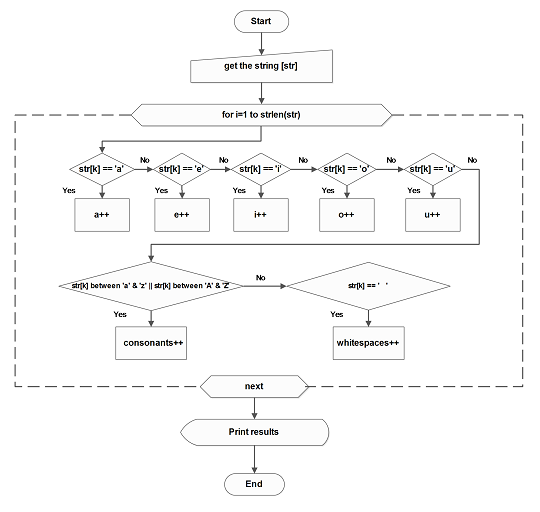
Program Code – Program to Count Frequency of Vowels
/*Read a sentence and print frequency of each vowels and the total count of consonants */
#include <stdio.h>
#include <conio.h>
int main()
{
char str[100];
int a,e,i,o,u,consonant,whitespace;
a = e = i = o = u = consonant = whitespace = 0;
int k;
/* Get the Sentence */
gets(str);
/* read the sentence */
for(k=0;k<strlen(str);k++)
{
if(str[k]== 'a')
{
a = a+1;
}
else if(str[k] == 'e')
{
e = e +1;
}
else if(str[k] == 'i')
{
i = i +1;
}
else if( str[k] == 'o')
{
o = o + 1;
}
else if(str[k] == 'u')
{
u = u+1;
}
else if((str[k] >= 'a' && str[k] <= 'z')||(str[k] >= 'A' && str[k] <= 'Z'))
{
consonant ++;
}
else if (str[k] == ' ')
{
whitespace++;
}
}
printf("a :%d\n",a);
printf("e :%d\n",e);
printf("i :%d\n",i);
printf("o :%d\n",o);
printf("u :%d\n",u);
printf("Consonant :%d\n",consonant);
printf("whitespace :%d\n",whitespace);
/* count vowels and consonants */
/* print the results */
system("PAUSE");
return 0;
}
Apart from being a string program, we used built-in functions from C standard library to get string input (gets) and compute the length of the given input string (strlen).
Output
The output of the program is given below. When the sentence ” A large reptile” is entered, the program reads all the vowels, consonants and white spaces and print the results.
ENTER A WORD OR A SENTENCE:
A large reptile
_____________________________________________
a :1
e :3
i :1
o :0
u :0
CONSONANTS :8
WHITESPACES :2