The C graphic header file has a builtin function to draw various geometric shapes such as lines, circles, polygons, and quadrilaterals. In this article, you will learn to draw a rectangle using C programming graphics support.
Problem Definition
To draw the rectangle using C graphics support, we write a program that does the following:
- Declare all graphic variables including graphic driver.
- Initialize all graphic variables.
- Initialization of graph and set path to graphic library support in C compiler.
- Set the line style for rectangle.
- Draw the rectangle.
- Close the graph.
Source Code – Draw Rectangle
/* Program to draw a rectangle using C graphic support */ #include <stdio.h> #include <conio.h> #include <graphics.h> #include <stdlib.h> int main() /* Main function begins */ { /* Declare the graphic variables */ int gdriver, gmode, userpattern; int left, right, top, bottom; /* Initialize the graphic driver to autodetect the graphic drivers */ gdriver = DETECT; userpattern = 1; /* Initialization of graph and path set to graphic interface */ initgraph(&gdriver, &gmode, "D:\\TURBOC3\\BGI"); /* Set the line style */ setlinestyle(SOLID_LINE,userpattern,3); /* Draw the line */ left = 100; top = 80; bottom = 220; right = 350; rectangle(left, top, right, bottom); /* close the graph */ getch(); closegraph(); return 0; }
The most important code is setting dimensions of the rectangle which draw the shape.
left = 100; top = 80; bottom = 220; right = 350;
Each variable is a corner point of the rectangle that defines the length and breadth of the sides of the rectangle. The left is 100 px in x-axis direction, top is 80 px in y-axis direction, the bottom is 220 px in x-axis and the right is 350 px in y-axis direction.
The (right – left) is 250px ( longer side) and the (bottom – top) is 120 px (shorter side) of the rectangle.
Output
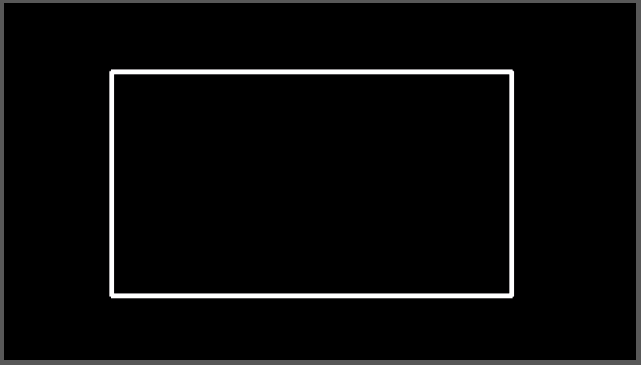