The C programming language allows drawing graphic shapes such as lines, rectangle, circles and so on. In this article, you will learn to write a program that draws a line on the console.
This program is written using turbo C++ compiler installed on a windows 7 64-bit PC.
Problem Definition
The program uses the C graphic header files and uses the builtin functions to draw a line on the screen. The important steps in this program are:
- Declare the graphic driver and graphic mode variable.
- Initialize the graphic driver
- Initialize the graph and set the path to the graphic support.
- Draw the line
- Close the graph.
Each of these steps are specific lines of codes in the program.
Source Code – C Line Drawing
/* Program to draw a line using C graphic support */ #include <stdio.h> #include <conio.h> #include <graphics.h> #include <stdlib.h> int main() /* Main function begins */ { /* Declare the graphic variables */ int gdriver, gmode, userpattern; int x, y, x_max, y_max; /* Initialize the graphics driver to autodetect the graphics drivers */ gdriver = DETECT; userpattern = 1; /* Initialization of graph and path set to graphics interface */ initgraph(&gdriver, &gmode, "D:\\TURBOC3\\BGI"); /* Set the line style */ setlinestyle(DOTTED_LINE,userpattern, 3); /* Draw the line */ x = 100; y = 70; x_max = 350; y_max = 70; line(x, y, x_max, y_max); /* close the graph */ getch(); closegraph(); return 0; }
Output
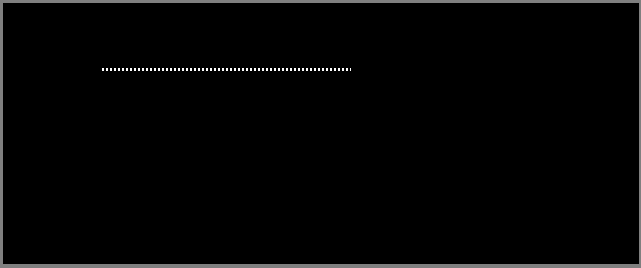