The switch-case
statements are similar to if-else-if
construct – a multiple decision maker. It allows you to choose a constant (a switch) that matches with a case (constant) and executes all statements that follow the matched case.
The main difference between if-else-if construct and switch-case is that the if-else-if uses multiple conditions at each level. The switch-case on the other hand, use only constants to choose a case and execute statements. The general format for switch-case is given below.
switch(ch)
{
case 1:
statement1;
statement2;
break;
case 2:
statement3;
statement4;
break;
default:
statement5;
break;
}
Points to Remember
- The switch can only take integer constants, or character constants. Do not use float, expression or strings.
- Value that follow case must be integer constant or character constant, must not be float, expression or strings.
- Each case is terminated using break statement.
When to use if-else-if and switch-case?
It is very hard to tell when to use switch-case or if-else-if constructs, but when you have many expressions to test at different level then if-else-if is suitable.
The switch-case is like choosing from a menu, you make a choice with a single character and if a case matches it executes the statements under that case. If no case matches then the default case executes its statements.
For example,
if (num > 100)
{
do something;
}
else if(num == 100)
{
do something;
}
else
{
do something;
}
The above if-else-if code can be implemented using switch-case, but without conditions and only with a constant value.
switch(ch)
{
case 1: // num > 100
do something;
break;
case 2: // num == 100
do something;
break;
default: // num < 100
do something;
break;
}
Both are similar, but, for the switch-case
we need to write conditions for each case. Hence, if-else-if
is more clear and better choice.
Graphical Representation of switch-case
The graphic representation of a program is necessary to understand the algorithm of a C++ program. You can use this diagram to represent a switch-case statement in the program.
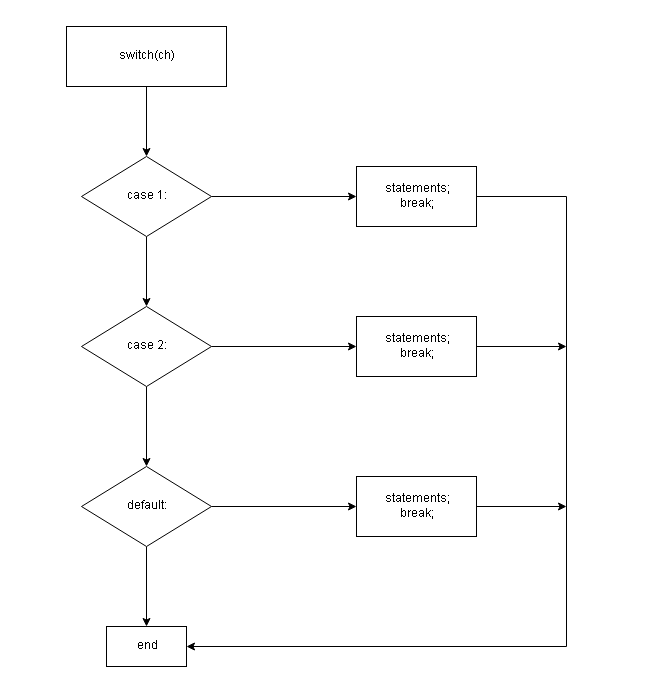
Example Program:
// Program to display weekday using switch-case
#include <cstdlib>
#include <iostream>
using namespace std;
int main()
{
//Variable declaration
int ch;
//Read input value
cout << "Enter a Number between 1 to 7 to Display Weekday:";
cin >> ch;
//using switch-case to display weekday
switch(ch)
{
case 1:
cout << "It is Monday" << endl;
break;
case 2:
cout << "It is Tuesday" << endl;
break;
case 3:
cout << "It is Wednesday"<< endl;
break;
case 4:
cout << "It is Thrusday" << endl;
break;
case 5:
cout << "It is Friday" << endl;
break;
case 6:
cout << "It is Saturday" << endl;
break;
case 7:
cout <<"It is Sunday" << endl;
break;
default:
cout << "Wrong Input ! Enter number between 1-7" << endl;
break;
}
system("PAUSE");
return EXIT_SUCCESS;
}
Output:
Enter a Number between 1 to 7 to Display Weekday:5
It is Friday