The C program for matrix manipulation performs basic matrix operations upon receiving the values for two matrices from the user. The program does addition, subtraction, multiplication, and transpose of a matrix.
We wrote the program using Dev-C++ version 4 compiler installed on Windows 7 64-bit. You can work with other standard C compilers after modifying the source code of the program. This is necessary to compile an error-free program.
You must be familiar with the concepts of a matrix and its operations to understand this example. See the problem definition section for a brief introduction to these concepts. Also, learn following C programming concepts before you practice this example.
- C Reading Input Values
- C Arithmetic Operators
- C Variables and Constants
- C Arrays
- C Flow Control Structures
- C For Loop
- C Functions
Problem Definition
Suppose you are given two matrices, then you can perform following simple operations on them.
- Addition
- Subtraction
- Multiplication
- Transpose
The program uses only square matrices for all its operations. For example, if and
are two matrices then,
// A Matrix
//B Matrix
// Transpose of A Matrix
// Addition of A and B matrix
//Subtraction of A and B matrix
// Multiplication of A and B matrix
More about the operations ..
- In multiplication of two matrices A and B, the row in A multiply all elements in column in B and the result is added to get each element of the output matrix. You do this for all rows and columns.
- In transpose of matrix, the row becomes the column and the column becomes the row, in the resultant matrix.
- The addition and subtraction of matrices are straight-forward operations.
Flowchart Matrix Manipulation Program
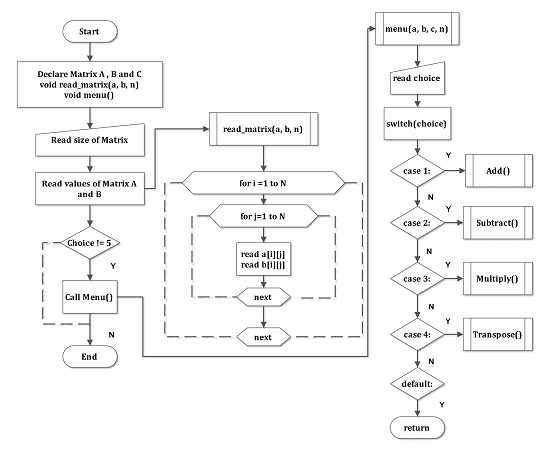
Program Code – Matrix Manipulation Program
/* PROGRAM IN C TO MANIPULATE MATRIX */
#include <stdio.h>
#include <conio.h>
#define N 10
main()
{
int a[10][10],b[10][10];
int c[10][10];
int i,j,k,n,choice;
void read_matrix(int a[N][N],int b[N][N],int n);
void menu(int a[N][N],int b[N][N],int c[N][N],int n);
/* Get the Size of the Matrices */
printf("ENTER SIZE OF MATRIX:");
scanf("%d",&n);
/* Read Matrices values */
read_matrix(a,b,n);
i=0;
while(choice !=5)
{
menu(a,b,c,n);
i++;
}
system("PAUSE");
return 0;
}
void menu(int a[N][N],int b[N][N],int c[N][N],int n)
{
int i,j,k,choice;
printf("ENTER A CHOICE:\n");
for(i=0;i<30;i++)
printf("_");printf("\n\n");
printf("1.ADDITION\n");
printf("2.SUBTRACTION\n");
printf("3.MULTIPLICATION\n");
printf("4.TRANSPOSE\n");
printf("5.QUIT\n")
for(i=0;i<30;i++)
printf("_");printf("\n\n");
scanf("%d",&choice);
i=0;
switch(choice)
{
case 1:
for(i=0;i<n;i++) {
for(j=0;j<n;j++) {
c[i][j] = a[i][j] + b[i][j];
}
}
/* Print Result */
system("cls");
printf("RESULT\n");
for(i=0;i<n;i++) {
for(j=0;j<n;j++) {
printf("%d\t",c[i][j]);
}
printf("n");
}
for(i=0;i<30;i++)
printf("*");
printf("\n\n");
break;
case 2:
for(i=0;i<n;i++) {
for(j=0;j<n;j++) {
c[i][j] = a[i][j] - b[i][j];
}
}
/* Print Result */
system("cls");
printf("RESULT\n");
for(i=0;i<n;i++) {
for(j=0;j<n;j++) {
printf("%d\t",c[i][j]);
}
printf("\n");
}
for(i=0;i<30;i++)
printf("_");printf("\n\n");
break;
case 3:
for(i=0;i<n;i++) {
for( j=0;j<n;j++) {
for( k=0;k<n;k++) {
c[i][j] = c[i][j] + a[i][k] * b[k][j];
}
}
}
/* Print the result */
system("cls");
printf("Result\n");
for( i=0;i<n;i++) {
for(j=0;j<n;j++) {
printf("%d\t",c[i][j]);
}
printf("\n");
}
for(i=0;i<30;i++)
printf("_");printf("\n\n");
break;
/* Transpose Matrix -A */
case 4:
for(i=0;i<n;i++) {
for(j=0;j<n;j++) {
c[i][j] = a[j][i];
}
}
/* Print Result */
system("cls");
printf("*RESULT*\n");
for(i=0;i<n;i++) {
for(j=0;j<n;j++) {
printf("%d\t",c[i][j]);
}
printf("\n");
}
for(i=0;i<30;i++)
printf("_");printf("\n\n");
break;
default: exit(0);
}
}
/* Read Matrix a and b*/
void read_matrix(int a[N][N],int b[N][N],int n) {
int i,j;
printf("READ MATRIX A:\n\n");
printf("ENTER MATRIX VALUES ROW WISE:\n\n");
for(i=0;i<n;i++) {
for(j=0;j<n;j++) {
scanf("%d",&a[i][j]);
}
}
/* Print Matrix A */
printf("*Matrix-A*\n");
for(i=0;i<n;i++) {
for(j=0;j<n;j++) {
printf("%d\t",a[i][j]);
}
printf("\n");
}
for(i=0;i<30;i++)
printf("*");printf("\n\n");
/* READ MATRIX B */
printf("READ MATRIX B:\n\n");
printf("ENTER MATRIX VALUES ROW WISE:\n\n");
for(i=0;i<n;i++) {
for(j=0;j<n;j++) {
scanf("%d",&b[i][j]);
}
}
/*Print Matrix-B */
printf("*Matrix-B*\n");
for(i=0;i<n;i++) {
for(j=0;j<n;j++) {
printf("%d\t",b[i][j]);
}
printf("\n");
}
for(i=0;i<30;i++)
printf("*");printf("\n\n");
}
Output
ENTER SIZE OF MATRIX:2
READ MATRIX A:
ENTER MATRIX VALUES ROWWISE:
1 2
4 2
*MATRIX-A*
1 2
4 2
********************************
READ MATRIX B:
ENTER MATRIX VALUES ROWWISE:
1 4
1 1
*MATRIX-B*
1 4
1 1
*****************************
ENTER A CHOICE:
___________________________
1.ADDITION
2.SUBTRACTION
3.MULTIPLICATION
4.TRANSPOSE
5.QUIT