In the previous article, you learn to draw a polygon shape with N points. In this article, you will learn to draw a polygon and fill color in it using graphics functions.
Problem Definition
If you want to draw a polygon shape with N points, where a point is represented using coordinates, then use
function from graphics.h header file of C language.
But we want to draw a polygon and fill some color in it. To write a program that draws a polygon and fill color or pattern follow the steps given below:
- Declare all graphics and non-graphics variables.
- Initialize the variables.
- Initialize the graph while a path points to the graphics files – BGI.
- Initilize the polygon array where each pair is a point in the polygon.
- Set fill style and color for the polygon shape.
- Draw the polygon.
- Close the graph.
Program Code – fillpoly ( ) with Color
/* C Program to draw a Polygon and fill color in C language */ #include <graphics.h> #include <stdio.h> #include <stdlib.h> #include <conio.h> int main() { /* Declaring a variable */ int gdriver, gmode; /* Polygon array to define points on the polygon shape */ int poly[10]; /* Initialize the variables */ gdriver = DETECT; /* Initialize the graph and set path to BGI files */ initgraph(&gdriver, &gmode, "D:\\TURBOC3\\BGI"); /* Polygon Points in Pairs */ poly[0] = 20; /* 1st vertex */ poly[1] = 100; poly[2] = 120; poly[3] = 140; /* 2nd vertex */ poly[4] = 240; poly[5] = 260; /* 3rd vertex */ poly[6] = 120; poly[7] = 320; /* 4th vertex */ poly[8] = poly[0]; poly[9] = poly[1]; /* The polygon does not close automatically, so we close it */ /* Set the fill style for the Polygon */ setcolor(getmaxcolor()); setfillstyle(SOLID_FILL, RED); /* Draw the Polygon */ fillpoly(5, poly); getch(); /* Close the graph */ closegraph(); return 0; }
Output – Fillpoly ()
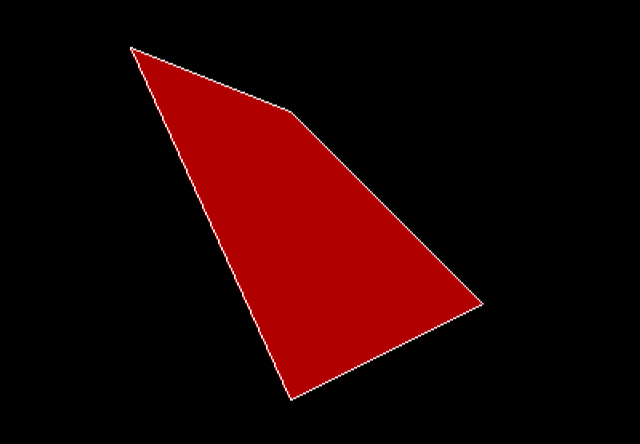