C programming allows to read or write files. In this program, a user will enter some text which will be stored in a file using file operations in C.
Learn C programming concepts, before you begin with this example.
Problem Definition
The program demonstrate the C language ability to write to file and it is achieved with the help of following C language features.
FILE – a file structure data type which is usually hidden from users.
fopen() – builtin function to open a file in buffer, so that you can read or write to file.
fprintf() – a print function to write to file from console.
fclose() – close the file and file pointer.
Program Source Code
Before you start executing the program, make sure you have created a file called demo.txt in the same directory where the program resides.
#include <stdio.h>
#include <stdlib.h>
int main()
{
char c[1000];
FILE *fptr;
fptr = fopen("demo.txt","w");
if(fptr == '\0')
{
printf("Error!!\n");
exit(1);
}
printf("Enter a Sentence:\n\n");
gets(c);
fprintf(fptr,"%s", c);
fclose(fptr);
system("PAUSE");
return 0;
}
Output
When run the program, it will ask for a sentence from user as input. See the diagram below, type the sentence you want to write to the file and press enter.
Enter a Sentence:
OMG ! This is a big red horse.
Press any key to continue . . .
You must go to the directory where the C program resides and open the file – demo.txt. The content of the console is written to the file successfully.
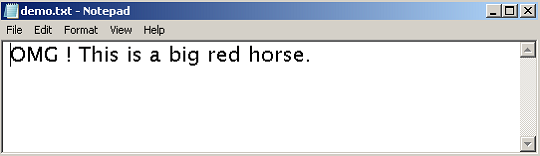