Variables are the most important element of any programming language. Variables hold values so that you can write expressions using them.You evaluate the expressions and again use a variable to store the results.
In JavaScript, variables are created using keyword “var”. There is no need to declare the type of the variable before assigning a value because as soon as you assign a value to the variable, the type is automatically decided.In this exercise, you will create an external JavaScript file called <span style="color:#cf2e2e" class="tadv-color">main.js</span>
and an HTML page called <span style="color:#cf2e2e" class="tadv-color">ex05.html</span>
and save it to a folder on your windows desktop (exercise-05). To know the difference between external and internal JavaScript files, go through exercise-03 and exercise-02.
JavaScript File
In this section, you will create a new text file, insert the example JavaScript code and save the file as <span style="color:#cf2e2e" class="tadv-color">main.js</span>
.
Step 1: Create a new folder on your windows desktop called “Exercise-05”.
Step 2: Create a new text file inside the “Exercise-05” folder and save it as main.txt.
Step 3: Open the main.txt file and insert the following JavaScript code.
var carname = "Hundai";
var carnumber = 2340;
var num = 10;
var n = 10;
var i = 0;
document.write("This is a car and it's Model is"
+ " " + carname + "");
document.write("The car number is " + " "
+ carnumber + "");
document.write("\n");
var txt1 = "What a very";
var txt2 = "nice day";
var txt3 = txt1 + " " + " " + txt2;document.write(txt3 + " ");
for (i = 0; i < n; i++)
{
num = num * 4;
}
document.write("Number=" + num);
Step 4: Save the file as <span style="color:#cf2e2e" class="tadv-color">main.js</span>
in “Exercise-05” folder and close it.
The variable names, carname
, txt1
, txt2
and txt3
are decided using the keyword “var
” and assigned a string value “Hyundai”. It automatically becomes a variable of type String.
Similarly, the variable carnumber
, num
and i
are declared using keyword “var
” and assigned an integer value, so they automatically become variables of type integer.
We will discuss more JavaScript data types later.
The JavaScript expressions use variables and in the example JavaScript, variables txt3
stores the result of the concatenation of two strings – txt1
and txt2
. The Variable num
is multiplied with 4 repeatedly until the loop exits and the final result is stored in variable num
itself.
Create an HTML Page
Assuming that you have done all the steps mentioned in the previous section, in this section, you will create an HTML page called ex05.html and link JavaScript file main.js to the HTML document.
Step 1: Create a new text file called <span style="color:#cf2e2e" class="tadv-color">ex05.txt</span>
in the folder <span style="color:#cf2e2e" class="tadv-color">Exercise-05</span>
on your windows desktop.
Step 2: Open the <span style="color:#cf2e2e" class="tadv-color">ex05.txt</span>
file and insert the following HTML code and save it as <span style="color:#cf2e2e" class="tadv-color">ex05.html</span>
.
<!DOCTYPE html>
<html>
<head>
<title>
Basic Javascript: Exercise-05:Variable Declarations
</title>
</head>
<body>
<p>Javascript Variable, String Concatenation and Loop</p>
</body>
</html>
Step 3: Add the following script to link the JavaScript file <span style="color:#cf2e2e" class="tadv-color">main.js</span>
to the HTML page ex05.html between <head> ... </head>
section.
<script type="text/JavaScript" src="main.js"></script>
Output to Browser
You will see the following output in the browser.
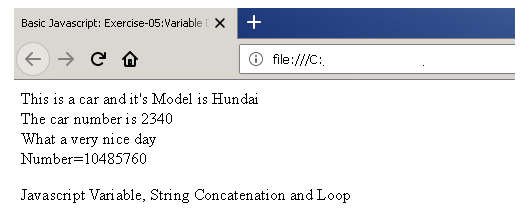