JavaScript pop-up boxes are very important part of web applications. JavaScript programming allows 3 types of pop-up boxes or dialog boxes.
- Alert boxes
- Confirm boxes
- Prompts
Alert Boxes
Alert boxes give immediate feedback to the user. There are many reasons to provide an alert message to users.
- To give them some information
- Give warning
- Error reporting
- A message about success
When a user gets an alert, he or she can click “OK” to continue working on another part of the browser. As long as an alert is displayed, it forces users to read the messages and other browser components are hidden.
Syntax:alert (“This is an example alert”);
Confirm Boxes
When you want users to accept something use a confirm box. This box is used for critical operations like deleting a file, saving a file, etc. It requires user confirmation to avoid accidental change or deletion.
Confirm boxes have two options – OK and Cancel. The OK returns “true” and Cancel returns “false”.
Syntax:confirm (“Do you wish to proceed?”);
Prompts
A prompt allows users to provide input for manipulation. When users create events like clicking a button, page load, etc. A prompt will pop-up and ask for user input. Users can click OK after providing their inputs.
Syntax:prompt (“Enter a number”,” “);
Example Program – Alert, Confirm and Prompt
In this example program, we will create a JavaScript file called alert.js and an HTML document called Ex08.html and verify the output for three JavaScript pop-up boxes.
Before you start the exercise, create a new folder – Exercise 08 and save your JavaScript file and the HTML page inside this folder.
JavaScript File – Alert.js
The step-by-step instruction to create the JavaScript file – Alert.js is given below.
Step 1: Open a notepad and save it as Alert.txt file.
Step 2: Insert the following code in Alert.txt and save it as Alert.js inside the Example 08 folder.
function alert_box()
{
alert("This is an alert box!");
}
function confirm_box()
{
var r = confirm("Press a button");
if(r == true)
{
alert("That was OK");
}
else
{
alert("That was cancel");
}
}
function prompt_box()
{
var name = prompt("Please enter your name","Name");
if(name!=null && name!= " ")
{
document.write("Hello" + " " + name
+ " " + "Thank You!");
}
}
Step 3: Close the file.
Now, it’s time to create an HTML page and link the JavaScript file to the HTML page.
Note:- You can also create an inline script within the HTML document.
The HTML file-Ex08.html
The step-by-step instructions to create an HTML file-Ex08.html is given below.
Step 1: Open a notepad and save it as Ex08.txt.
<!DOCTYPE html></span>
<span style="font-family: andale mono, monospace;"><html></span>
<span style="font-family: andale mono, monospace;"><head></span>
<span style="font-family: andale mono, monospace;"><title>
</span>
<span style="font-family: andale mono, monospace;"> Show Alert
</span>
<span style="font-family: andale mono, monospace;"></title></span>
<span style="font-family: andale mono, monospace;"></head></span>
<span style="font-family: andale mono, monospace;"><body></span>
<span style="font-family: andale mono, monospace;"> <p><em> This is demo for showing JavaScript Pop up.</em><p>
<frameset>
</span>
<span style="font-family: andale mono, monospace;"> <legend>JavaScript Examples</legend></span>
<span style="font-family: andale mono, monospace;"> <input type="button" onclick="alert_box()"
value="show alert box"/></span>
<span style="font-family: andale mono, monospace;"> <input type="button" onclick="confirm_box()"
value="show confirm box"/></span>
<span style="font-family: andale mono, monospace;"> <input type="button" onclick="prompt_box()"
value="show prompt box"/>
</frameset></span>
<span style="font-family: andale mono, monospace;"></body></span>
<span style="font-family: andale mono, monospace;"></html>
Step 3: Add the following line of JavaScript code to link the HTML page with JavaScript file.
<!DOCTYPE html>
</span>
<span style="font-family: andale mono, monospace;"><html></span>
<span style="font-family: andale mono, monospace;"><head></span>
<span style="font-family: andale mono, monospace;"><title></span>
<span style="font-family: andale mono, monospace;"> Show Alert</span>
<span style="font-family: andale mono, monospace;"></title></span>
<span style="font-family: andale mono, monospace;"><script type="text/javascript" src="Alert.js"></script></span>
<span style="font-family: andale mono, monospace;"></head></span>
<span style="font-family: andale mono, monospace;"><body></span>
<span style="font-family: andale mono, monospace;"> <p><em> This is demo for showing JavaScript Pop up.</em><p></span>
<span style="font-family: andale mono, monospace;"><fieldset></span>
<span style="font-family: andale mono, monospace;"> <legend>JavaScript Examples</legend></span>
<span style="font-family: andale mono, monospace;"> <input type="button" onclick="alert_box()"
value="show alert box"/></span>
<span style="font-family: andale mono, monospace;"> <input type="button" onclick="confirm_box()"
value="show confirm box"/></span>
<span style="font-family: andale mono, monospace;"> <input type="button" onclick="prompt_box()"
value="show prompt box"/></span>
<span style="font-family: andale mono, monospace;"></fieldset></span>
<span style="font-family: andale mono, monospace;"></body></span>
<span style="font-family: andale mono, monospace;"></html>
Output to the Browser
The output of the program is given below. The HTML page shows three buttons one for each type of JavaScript pop-up box. When a user clicks the button, it will create an event called onclick(). As a result, the respective pop-up code is executed and output is displayed on the browser.
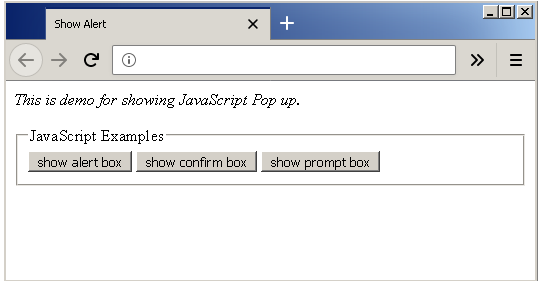
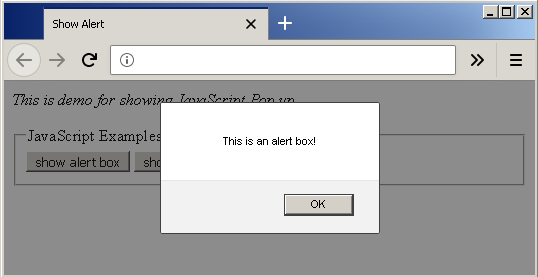
When a user clicks on the alert button when they see an alert – “This is an alert box”.
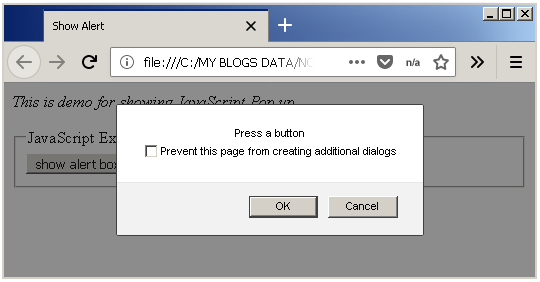
When a user clicks the second button- the Confirm box button, they get a pop-up with an OK and a Cancel.
The third button when clicked will display a prompt asking username as in above diagram. You need to enter and name and then click OK.
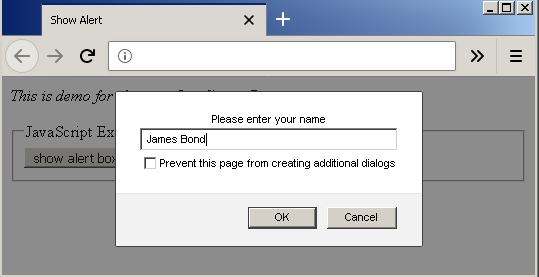
The following output will be displayed on the browser.
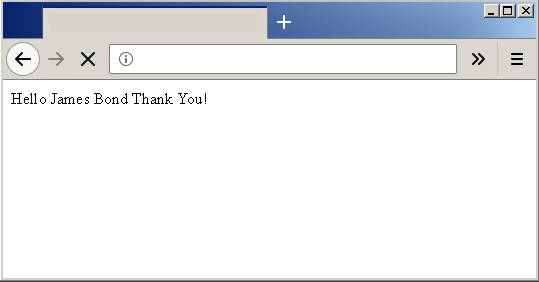