In the previous article, you learned about the objects and its properties.In this article, you will learn about nested objects, that is, objects with in another object. This is very important to create a list of objects.
Structure of nested objects
The nested objects are objects themselves becoming properties of another object. They way to create a nested object is not different from creating a simple object. Consider the following example.
var students = {
2457: {
name: "John dunn",
section: "A",
class: 10,
address: {
"building no": 12,
"street" : "parker lane",
"city" : "New York",
"country" : "USA"
};
};
2458: {
name: "Akbar ali",
section: "B",
class : 10,
address: {
"building no" : 17,
"street" : "rampur",
"city" : "new delhi",
"country" : "INDIA"
};
};
};
In the example above, the student
is the primary object and 2458
(student id) is a nested object in student
object. The address
object is nested inside the 2458
object.
Accessing the property in nested object
You can access the property of a nested object in the same way you would do for any ordinary JavaScript object.
You must specify the fully qualified path to that property in order to access that nested object property. There is a hierarchical relationship between primary and nested objects.
Consider the following example:
student.2458.address.city = "Mumbai";
In the example above, we accessed the student
object whole id is 2458
and whose city
is set to Mumbai
. In this way you can specify and access other properties at any level.
For example.
student.2458["name"] = "Akbar Ali Hansari";
In the example above, we changed the name
of the student
with id 2458
. The path to the property is shorter this time.
In this way you can do following operations on JavaScript nested objects.
- Create new properties.
- Update the value of a nested object property.
- Display nested object properties.
- Delete a nested object property.
Example Program: Nested Objects
<!DOCTYPE html>
<html>
<head>
<title>JavaScript - Nested Objects</title>
<meta charset = "utf-8">
</head>
<body>
<h3 id="result">Get output for creating property</h3>
<h3 id="result2">Get output for display property</h3>
<h3 id="result3">Get output for property delete</h3>
<button onclick="myNestedObject()">Click me!</button>
<script>
function myNestedObject(){
var students = {
2457: {
name: "John dunn",
section: "A",
standard: 10,
address: {
"building no": 12,
"street" : "parker lane",
"city" : "New York",
"country" : "USA"
}
},
2458: {
name: "Akbar ali",
section: "B",
standard: 10,
address: {
"building no" : 17,
"street" : "rampur",
"city" : "new delhi",
"country" : "INDIA"
}
}
};
/* Create a new property called age for student 2458 */
students[2458].age = 16;
document.getElementById("result").innerHTML =
students[2458].age;
/* Display a property */
document.getElementById("result2").innerHTML =
students[2458].address.city;
/* Delete Property */
delete students[2457].section;
document.getElementById("result3").innerHTML =
"Property deleted successfully";
}
</script>
</body>
</html>
Output – JavaScript Nested Objects
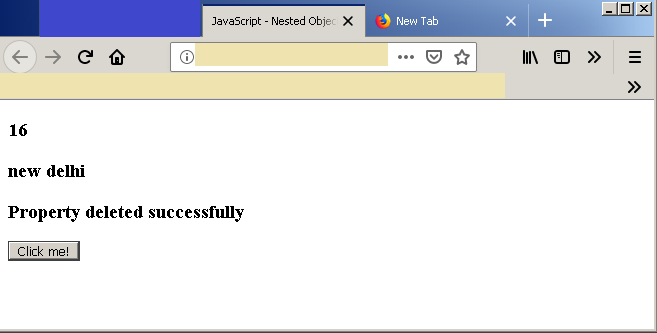