In this article, you will learn about basics building blocks of R programming such as constants, variables and vectors.
R programming uses various arithmetic operators to manipulate constants, variables and vectors.
Arithmetic operators in R
In R programming, the following are the arithmetic operators.
Operator | Meaning |
+ | addition |
– | subtraction |
* | multiplication |
/ | division |
^ | exponential |
%% | mod |
Apart from the above the R programming also use some math functions such as sqrt
() and abs()
. The sqrt() function returns square root of a number or vector and the abs() function gives absolute value of a variable or vector.
Constants in R
The constant is a number, or character that can be manipulated without assigning it a name. You can use R console like a calculator simply using the constants.
Consider following examples.
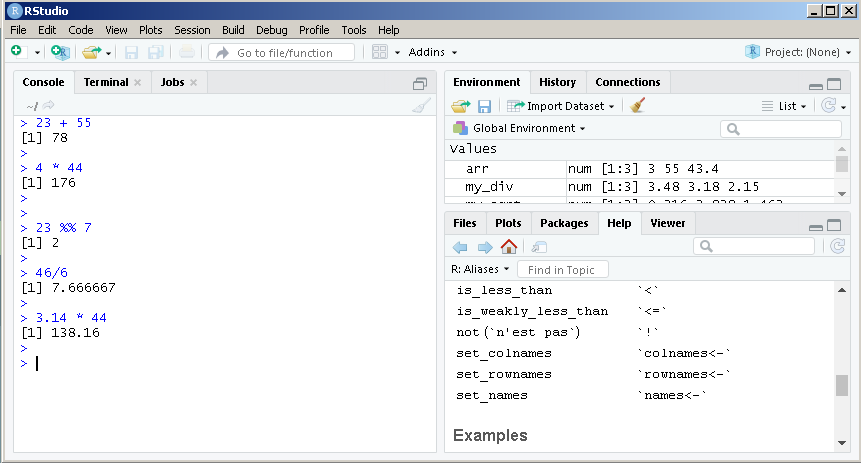
From the above figure, it is clear that you can use the R console as calculator. Simply type the operators and operands and you get the output. Note that this only works for numeric data.
Joining two strings would result in error.
> "Hello" + "World"
Error in "Hello" + "World" : non-numeric argument to binary operator
>
Note: If you want to clear the console press Ctrl + L on your keyboard.
Variables in R
Variables are used to store values that are used in expressions. The results of expressions are also stored in variables.
Variables can store different types of data such as numbers, strings, and vectors.
In R programming, you have to assign values to variables. In case, you are using R console then the traditional assignment operator (=) works with new assignment operators given below.
Assignment Operator | Meaning |
<-, <<-, = | Right to left assignment |
->, ->> | Left to right assignment |
Following are some examples of right to left variable assignment in R.
## Right to Left assignment
> A = 200
> A
[1] 200
>
> B <- 300
> B
[1] 300
>
> C <<- 400
Error: cannot change value of locked binding for 'C'
> B <<- 400
> B
[1] 400
> D <<- 500
In the example, the C <<- 400
failed because <-
and <<-
works differently. The operator <
– means “assign the left hand value to right hand variable”, however, C <<- 400
means “find the variable C in the current scope and then if found assign the value of 400” .
The variable C <<- 400
is not working because C is not defined earlier. But, B <<- 500
works as it is available in the current scope.
Let us see some more examples right to left of assignment
> 100 -> P
> P
[1] 100
> 200 ->> Q
> Q
[1] 200
>
Vectors in R
You can create vectors in R programming. These vectors can take many types of data such as strings, numbers, double, Boolean, etc.
To create a vector use following syntax.
> my_vector <- c(1,2,4,5, 34.2, "Hello", TRUE)
> my_vector
[1] "1" "2" "4" "5" "34.2" "Hello" "TRUE"
>
You can perform arithmetic operations with a vector like any ordinary variable. Consider the following example.
> c(20, 30, 50) * 5
[1] 100 150 250
>
In the example above, the number 5 is multiplied with each element of the vector.
Similarly, you can append an array with more elements.
> myVector <- c(27, 55, 33.2)
> z <- c(myVector, 100, 200)
> z
[1] 27.0 55.0 33.2 100.0 200.0
>
Using the above technique we have added two more elements 100 and 200 to the existing vector.
Arithmetic Between Two Vectors
You can even perform arithmetic operation between two vectors. Here is an example.
> a_vector <- c(100, 200, 300, 400) / c(2, 4, 6, 8)
> a_vector
[1] 50 50 50 50
Each element of the first vector is divided by the corresponding element of the second vector. Therefore, 100/2, 200/4, 300/6 and 400/8
give the output of 50 50 50 50
.
What if the second vector is smaller than the first one ?
> result_vector <- c(12, 44, 66, 22)/ c(2, 3)
> result_vector
[1] 6.000000 14.666667 33.000000 7.333333
>
If the second vector is smaller than the R programming “recycles” the second vector against the first vector. In the example above, 12/2, 44/2, 66/2 , and 22/3
resulted in 6.000000 14.666667 33.000000 7.333333.
Note how second vector repeat itself for the division.
If the second vector elements does not cover all elements in the recycling process,
then the arithmetic operation will fail.
> c_vector <- c( 122, 45, 55) * c(23, 44)
Warning message:
In c(122, 45, 55) * c(23, 44) :
longer object length is not a multiple of shorter object length
>
The error message clearly says that the shorter array must be multiple of longer vector
for recycling to happen.