Data types are fundamental to programming languages and they define the type of values get stored in a python variable. Here you will practice python data types which are similar to other programming languages, yet python don’t have strict type checking like C++, Java, etc. Strict type checking means the you need to declare the type of data before storing value in a variable. For example, int Num; Here ‘int’ means integer type.
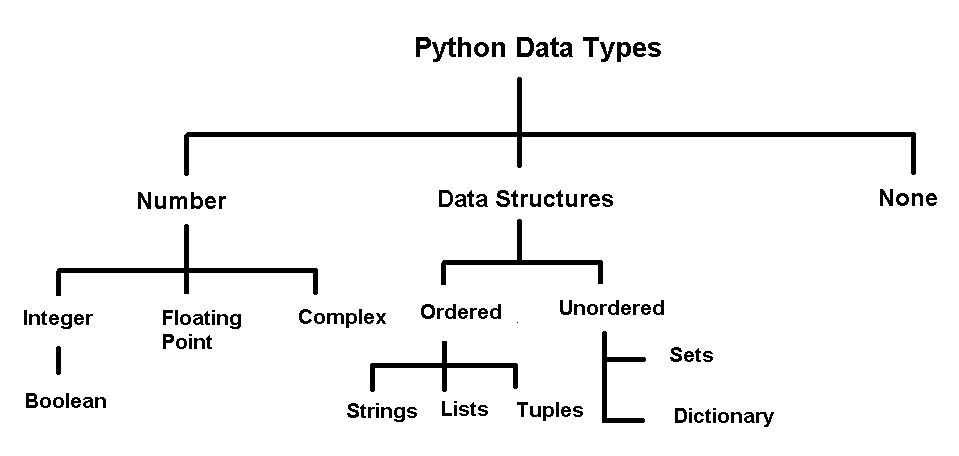
It is recommended that you try these problems on your own and then compare the results. May be you may come up with a better way to solve the problem.
Program 1: Write a program that store number data types , output the type and the data.
Solution:
# Filename : Number_type.py
# write a program that store number types, output the values and type
# number types are - integer, float , complex , and boolean
integer_type = 23
float_type = 344.66
complex_type = 3 + 2j
bool_type1 = True
bool_type2 = False
# Print values and their types
print(integer_type)
print(type(integer_type))
print(float_type)
print(type(float_type))
print(complex_type)
print(type(complex_type))
print(bool_type1)
print(type(bool_type1))
print(bool_type2)
print(type(bool_type2))
Output:
23
<class 'int'>
344.66
<class 'float'>
(3+2j)
<class 'complex'>
True
<class 'bool'>
False
<class 'bool'>
Program 2: Writing a program to store ordered data types such as strings, lists, and tuples and display their value. Also, output their type information.
Solution:
# Filename : ordered_data_type.py
# Writing a program to display values of ordered data type with type information
# python ordered types are string, list, and tuple
myString = "Himalayas"
myList = ["Milk","Banana", "Bread", "Chocolate", 400]
myTuple = (100, "Notesformsc", "ComputerScience")
# print the values and the type
print(myString)
print(type(myString))
print(myList)
print(type(myList))
print(myTuple)
print(type(myTuple))
Output:
Himalayas
<class 'str'>
['Milk', 'Banana', 'Bread', 'Chocolate', 400]
<class 'list'>
(100, 'Notesformsc', 'ComputerScience')
<class 'tuple'>
Program 3: Write a program to display third letter of the string “MOUNTAIN”.
Solution:
# Filename : Display_letter.py
# Writing a program to display third letter of the string "MOUNTAIN"
myString = "MOUNTAIN"
# index start at 0; therefore, third letter is index is 2
myLetter = myString[2]
# print the output
print("Third letter is",myLetter)
Output:
Third letter is U
Program 4: Write a program in Python to store a None type and display the results with its type information.
Solution:
# Filename : None_type.py
# Write a program that store None type and display the results with its type information.
# the None type store nothing, its not zero, not null, etc
myValue = None
print(myValue)
print(id(myValue))
Output:
None
1786346564
Program 5: Write a program that demonstrate that python sets cannot store duplicate values.
Solution:
# Filename : Sets_duplicate_value.py
# Write a program to demonstrate that python sets cannot store duplicate values.
mySet = {230, 500,100, 500, 670}
print(mySet)
print(type(mySet))
Output:
{100, 670, 500, 230}
<class 'set'>
Program 6: Write a program using dictionary to store records of 5 students and display them as output.
Solution:
# Filename : Student_record_dictionary.py
# Write a program using dictionary to store records of 5 students and display them as output.
firstStudent = {
"ID": 35,
"Name": "Ram",
"Subject":"Math",
"Class": 10,
}
secondStudent = {
"ID": 36,
"Name": "Megha",
"Subject":"Chem",
"Class": 10,
}
thirdStudent = {
"ID": 37,
"Name": "John",
"Subject":"Hist",
"Class": 11,
}
fourthStudent = {
"ID": 38,
"Name": "Lee",
"Subject":"Engl",
"Class": 12,
}
fifthStudent = {
"ID": 39,
"Name": "Geeta",
"Subject":"Math",
"Class": 12,
}
# Display student data
print("ID","Name","Section","Class")
print(firstStudent["ID"],firstStudent["Name"],firstStudent["Subject"],firstStudent["Class"])
print(secondStudent["ID"],secondStudent["Name"],secondStudent["Subject"],secondStudent["Class"])
print(thirdStudent["ID"],thirdStudent["Name"],thirdStudent["Subject"],thirdStudent["Class"])
print(fourthStudent["ID"],fourthStudent["Name"],fourthStudent["Subject"],fourthStudent["Class"])
print(fifthStudent["ID"],fifthStudent["Name"],fifthStudent["Subject"],fifthStudent["Class"])
Output:
ID Name Section Class
35 Ram Math 10
36 Megha Chem 10
37 John Hist 11
38 Lee Engl 12
39 Geeta Math 12