In this example, we will receive a two-dimensional array of numbers as input and the JavaScript function will find the largest in each sub-array and return the result in a new array.
The program takes following steps to compute the results:
- Receive an array with many sub-arrays.
- Set a maximum number variable.
- Loop through each sub-array,compare it with maximum and if the array number greater than maximum then get that array number as new maximum.
- Push the new maximum from the sub-array into a new result array.
- Repeat the above two steps for all sub-arrays.
- Display the resultant new array which contains maximum from all sub-arrays.
For example,
Suppose our array is following
[[17, 23, 25, 12], [25, 7, 34, 48], [4, -10, 18, 21], [-72, -3, -17, -10]]
Each of the sub-array has maximum and we will collect them in a new array. The result is as follows.
[25, 48, 21, -3]
Program Code: Find the Largest Number
<!DOCTYPE html>
<html>
<head>
<title>Find Largest number from Array</title>
<meta charset="utf-8">
<style>
main{
width:40%;
padding:2%;
margin:10% auto;
background:DodgerBlue;
text-align:center;
}
#in{
padding:10px;
width:150px;
background:whitesmoke;
margin:auto;
}
button{
padding:10px;
margin:10px;
}
</style>
</head>
<body>
<main>
<h1 id="out">Output here</h1>
<p>[[17, 23, 25, 12], [25, 7, 34, 48], [4, -10, 18, 21],
[-72, -3, -17, -10]]</p>
<button onclick="largestNumber()">Find Largest Number</button>
<button onclick="MyClear()">Clear</button>
</main>
<script>
function largestNumber() {
// You can do this!
var arr = [[17, 23, 25, 12], [25, 7, 34, 48],
[4, -10, 18, 21], [-72, -3, -17, -10]];
var maxNumber = -20;
var result = [];
for(let i = 0;i< arr.length;i++){
for(let j = 0; j < arr[i].length;j++){
if (arr[i][j] > maxNumber){
maxNumber = arr[i][j];
}
}
result.push(maxNumber);
maxNumber = -20;
}
document.getElementById("out").innerHTML = result;
}
</script>
</body>
</html>
Note that we did not receive input from a HTML control, but directly assigned it to a variable. The output is display in the browser though.
Output To Browser: Find the Largest Number
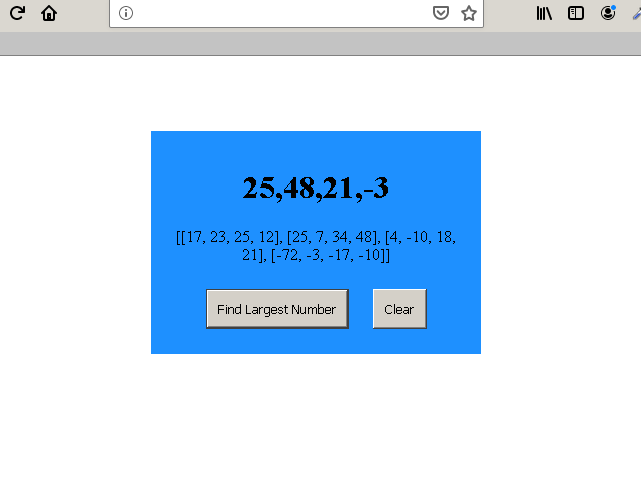