In this example, you will create a JavaScript function that will accept a string that contains multiple words and find the longest among those words and display the length of the string.
The program takes following steps to find the longest string.
- Accept the string in a function –
findLengthLongest()
. - Use JavaScript regex to extract each word from the string.
- Store the words in an array.
- Find length of each word in the array and copy in a variable called
max
. - At the end, max has the length of longest word.
- Display the result.
JavaScript Code: Find Longest Word in a String
function findLengthLongest() {
var str = document.getElementById("in").value;
var max = 0;
var regex = /\w+/gi;
var word = [];
word = str.match(regex);
for (let i = 0;i < word.length;i++){
if(word[i].length > max)
{
max = word[i].length;
}
}
var result = max.toString(10);
document.getElementById("out").innerHTML = result;
}
function MyClear(){
document.getElementById("in").value = "";
}
Note that we are accepting values from textbox
control and displaying result in a H1
element. To find the length, we use the .length
function and loop through each word of the array. Each time a greater length word if found, update the variable max
.
Program Code: Find Longest Word
<!DOCTYPE html>
<html>
<head>
<title>Find Longest Word</title>
<meta charset="utf-8">
<style>
main{
width:40%;
padding:2%;
margin:10% auto;
background:DodgerBlue;
text-align:center;
}
#in{
padding:10px;
width:150px;
background:whitesmoke;
margin:auto;
}
button{
padding:10px;
margin:10px;
}
</style>
</head>
<body>
<main>
<h1 id="out">Output here</h1>
<input type="text" id="in" /><br/>
<button onclick="findLengthLongest()">Find Longest Word</button>
<button onclick="MyClear()">Clear</button>
</main>
<script>
function findLengthLongest() {
var str = document.getElementById("in").value;
var max = 0;
var regex = /\w+/gi;
var word = [];
word = str.match(regex);
for (let i = 0;i < word.length;i++){
if(word[i].length > max)
{
max = word[i].length;
}
}
var result = max.toString(10);
document.getElementById("out").innerHTML = result;
}
function MyClear(){
document.getElementById("in").value = "";
}
</script>
</body>
</html>
Output To Browser: Find Longest Word
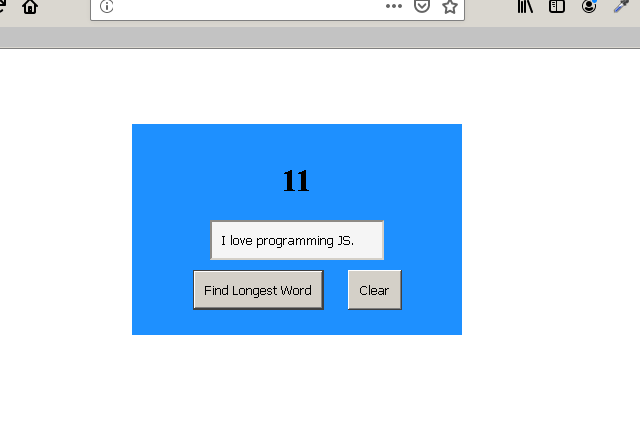