JavaScript arrays can hold different types of data unlike other programming languages like C, C++, and Java. In this article, you will create a simple array and store elements in the array.
Structure of array declaration
To declare an array in JavaScript use following syntax.
arrayName = []; //declare an empty array
arrayName = [23, "Car", 45.66, 'B']; //Assign values later
Or
var arr = [34,56,77,2,6];
JavaScript push() and pop() method
You can use JavaScript arrays like a stack. You can push element using the push() method or delete an element from the array.
Elements from array are pushed or deleted from the rear end of the array. You can consider the rear end of the array as top of stack.
var arr = [44,55,66,77];
// calling push function to insert 88
arr.push(88);
// calling push function to insert 100
arr.push(100);
// display the array
console.log(arr);
// delete an element using pop() function
arr.pop();
// display array
console.log(arr);
Two Dimensional Array
JavaScript allows you to create a multidimensional array. The two dimensional array has rows and columns. To create a two dimensional array use following syntax.
var arrTwoDimensional = [ [2,5],[3,6],[,4,8] ];
arrTwoDimensional.push([5,10]);
Example Program:
<!DOCTYPE html>
<html>
<head>
<title>JavaScript - Array Stack</title>
<meta charset = "utf-8">
</head>
<body>
<script>
var arr = [44,55,66,77];
// calling push function to insert 88
arr.push(88);
// calling push function to insert 100
arr.push(100);
// display the array
document.write(arr + "<br>");
// delete an element using pop() function
arr.pop();
// display array
document.write(arr + "<br>");
var arrtwoDimensional = [[2,3],[4,5],[6,7]];
arrtwoDimensional.push([8,9] + "<br>");
document.write(arrtwoDimensional);
</script>
</body>
</html>
Output – JavaScript Arrays
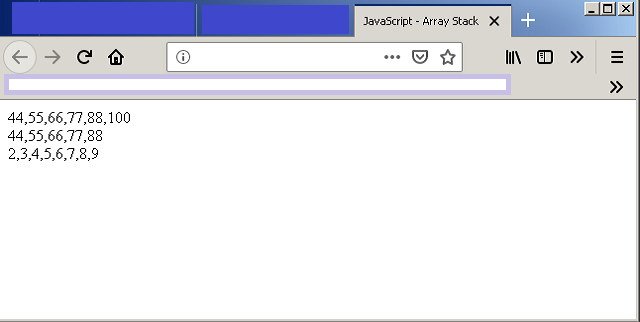