JavaScript objects are variables that hold multiple types of data. The objects represents real life or abstract ideas using properties and methods. In this article, you will learn to create a JavaScript object and access its members.
JavaScript Object Concept
Suppose you want to represent a car in you program. To represent a car object you must describe its properties and behaviors with methods or functions.
For example, if the car object is red in color. You can create property
called color
and give a value called
red
. Similarly, you can create function start()
and stop()
to describe the behavior of the car object.
JavaScript Object Structure
To create an object use the following examples:
var myCar = {
"Type: "Toyota",
"Model": "23VXV",
"Wheel": 4,
"Color": "Red",
"Price" : 2000000
};
You can describe object property without making it a string like above example. Consider the following example:
var student = {
firstName : "Ram",
lastName : "Kumar",
Roll Number: 1232,
Course : "BSc",
Major : "Computer Science"
}
Accessing Object Property
There are two ways to access the object property.
- Use the (.) dot notation
- Use the ([ ]) bracket notation.
Example of dot notation is as follows: The object name is student
and property name is Major
.
student.Major;
For multiple words property use [ ] bracket notation to access the object property.
student["Roll Number"];
How to update a JavaScript object property ?
If the object is available and has a property with some value. Then use following method to update that value.
student.Major = "Mathematics";
The above code will set a new value for student object's
property called Major
.
How to delete a JavaScript object property ?
Sometimes it is necessary to delete a JavaScript object property. You can delete the property using following statement;
delete student.Course;
Example Program: Objects
In this example, we will create and object called student
, access a property, change value of another property and delete a property of object student.
<!DOCTYPE html>
<html>
<head>
<title>JavaScript - Objects</title>
<meta charset = "utf-8">
</head>
<body>
<script>
/* Creating a new object */
var student = {
"firstName":"Peter",
"lastName":"Pan",
"roll No": 2367,
"course": "BSc",
"major": "Computer Science"
}
/* Display a property value */
document.write("First Name = " + student.firstName + "");
/* Update a property value */
student["roll no"] = 5555;
/* Delete a property */
delete student.course;
/* Creating a new property */
student.subject = "MSc";
document.write("Subject=" + student.subject + "<br>");
document.write("Roll No=" + student["roll no"]+ "<br>");
</script>
</body>
</html>
Output – JavaScript Objects
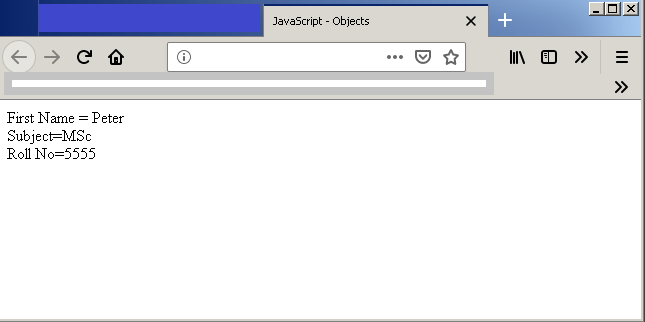