Earlier in the previous articles, you learned about objects and nested objects. In this article, you will learn about array of object. This kind of representation is known as JavaScript Object Notation(JSON) where objects are stored in key: value pair or arrays.
The JSON is open standard to store and transmit data objects between applications such as AJAX, etc.
This exercise has three parts.
- Create an array of objects.
- Write a function with parameters to access the list.
- Use
HTML
button to callJavaScript
function to display the content of the object.
JavaScript Code: Create an Array of Objects
In this section we will write the required JavaScript
code to create an array of object. The objects will be employees
with their official details.You may put this code in between <body> ...</body>
tag of the HTML
page.
var list = [
{
"firstName": "Kiran",
"lastName" : "Kumar",
"salary" : 25000
},
{
"firstName": "Mohan",
"lastName" : "Srivastav",
"salary" : 20000
},
{
"firstName": "Mary",
"lastName" : "Kom",
"salary" : 23000
}
];
The list
array contains objects employees with following properties – firstName
, lastName
, and salary
.
Function To Access the Object Property
Now, we can write a JavaScript function to access the property of the objects. We need to check following things before we could access the property.
- Check if the employee exist or not
- If yes, then proceed to display the content of the property supplied in the function parameters.
- If no, display “No such employee found!”
function lookup(num, prop){
for(var i=0;i<3;i++)
{
if(num == i)
{
document.getElementById("demo").innerHTML =
list[num][prop];
break;
}
else
{
document.getElementById("demo").innerHTML =
num + " " + i + " " +"No such contact found";
}
}
}
The function <span style="color:#cf2e2e" class="tadv-color">lookup(num, prop);</span>
takes two arguments.
- The index of the object to be accessed.
- The property name whose value you want to display.
HTML Code: Display the Object Property Value
It is time to create the required HTML elements that our JavaScript code will access to display the object property value.
<p id="demo">Output Here</p>
<button onclick="lookup(1, 'firstName')">Display</button>
We only created a button
and a paragraph
element in HTML
. When the user clicks the button, it will fire the JavaScript
function and output is displayed in the paragraph.
Complete Program Code: Array of Objects
<!DOCTYPE html>
<html>
<head>
<title>Array of Objects</title>
<meta charset="utf-8">
</head>
<body>
<p id="demo">Output Here</p>
<button onclick="lookup(1, 'firstName')">Display</button>
<script>
var list = [
{
"firstName": "Kiran",
"lastName" : "Kumar",
"salary" : 25000
},
{
"firstName": "Mohan",
"lastName" : "Srivastav",
"salary" : 20000
},
{
"firstName": "Mary",
"lastName" : "Kom",
"salary" : 23000
}
];
function lookup(num, prop){
for(var i=0;i<3;i++)
{
if(num == i)
{
document.getElementById("demo").innerHTML =
list[num][prop];
break;
}
else
{
document.getElementById("demo").innerHTML =
num + " " + i + " " +"No such contact found";
}
}
}
</script>
</body>
</html>
Output in Browser: Array of Objects
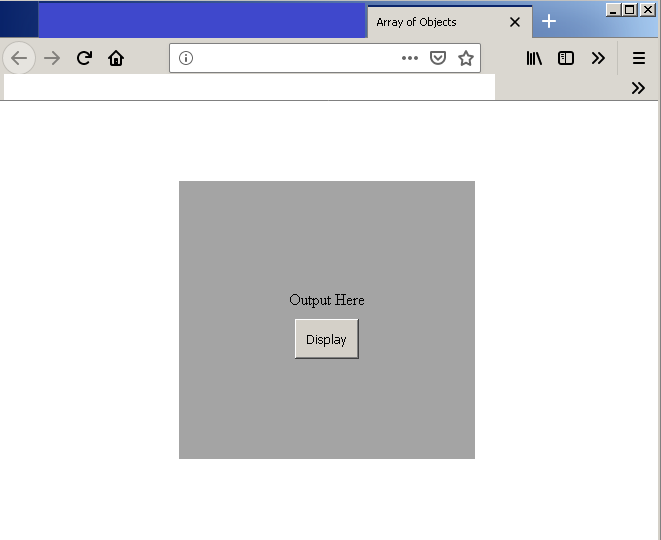