The python operators are used to evaluate expressions. An expression consists of values and operators which always evaluates to a single value.
The python operators are classified in following types.
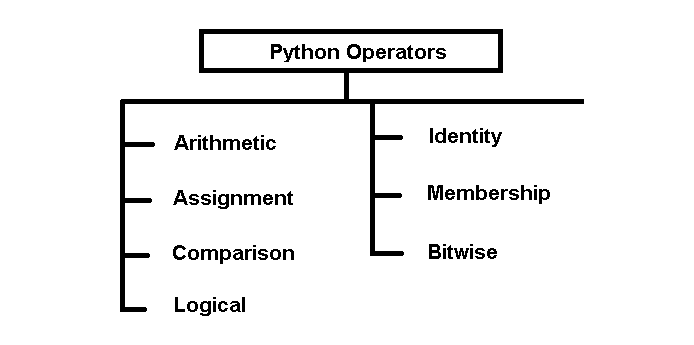
Program 1: Write a program to demonstrate the working of python arithmetic operator.
Solution:
#Filename : Arithmetic_Demo.py
#Write a program to demonstrate the working of python arithmetic operator.
#plus
num1 = 23
num2 = 56
result = num1 + num2
print("Addition",result)
#minus
num1 = 321
num2 = 100
result = num1 - num2
print("Subtraction",result)
#multiplication
num1 = 24
num2 = 10
result = num1 * num2
print("Multiplication",result)
#division
num1 = 59
num2 = 3
result = num1 / num2
print("Division",result)
#floor division
num1 = 100
num2 = 31
result = num1 // num2
print("Floor Division",result)
#exponential
num1 = 3
num2 = 2
result = num1 ** num2
print("Exponential",result)
Solution:
Addition 79
Subtraction 221
Multiplication 240
Division 19.666666666666668
Floor Division 3
Exponential 9
Program 2: Write a program to demonstrate the working of python assignment operators.
Solution:
File name: Assignment_demo.py
# simple assignment
x = 10
y = 20
z = 0
# compound addition assignment
x += 10
print("Compound Addition Assignment", x)